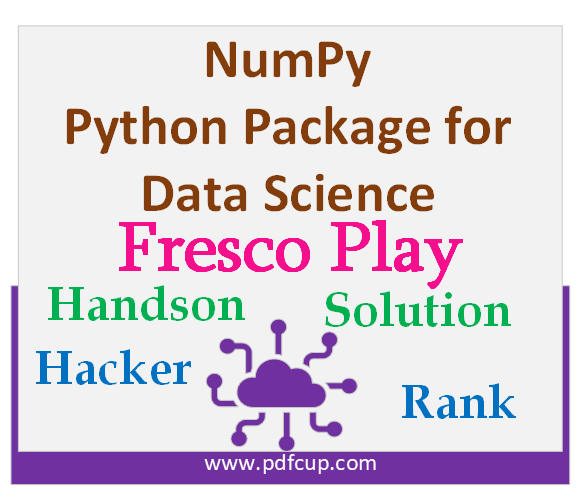
Kindly Note: If you had any problems, please comment below.
Fresco Play Numpy Hacker Rank Hands On
Lab 1 : Try it Out - Array Creation [Welcome to Array Creation - Hands-on]
Python "ndarray Array Creation" Hands-on
Solution: Python "ndarray Array Creation" Hands-on
import numpy as np
# Enter your code here. Read input from STDIN. Print output to STDOUT
def array_operations(l):
#Write your code below
x = np.array(l, dtype='float64')
print( type(x) )
print( x.ndim )
print( x.shape)
print(x.size)
if __name__ == "__main__":
l = list(map(int,input().split()))
array_operations(l)
Lab 2 : Try it Out - Determine Attributes [Welcome to Python Array - Determine Attributes - Hands-on]
Python Array "Determine Attributes" Hands-on
Solution : Python Array "Determine Attributes" Hands-on
import numpy as np
# Enter your code here. Read input from STDIN.
def array_attributes(l):
y = np.array(l)
x=y
# Type of the Array
print( type(x) )
# Dimensions of X
print( x.ndim )
#Shape of X
print( x.shape)
# Size of Y
print(x.size)
# Type of each data element of X
print(x.dtype)
#Number of Bytes occupied by each data element of X
print(x.itemsize)
if __name__=="__main__":
r=int(input())
l=[]
for i in range(r):
n = list(map(int,input().split()))
l.append(n)
array_attributes(l)
Lab 3: Try it Out - ndarray [Welcome to Python - ndarray - hands-on]
Python "ndarray" Hands-on
Solution: Python "ndarray" Hands-on
import numpy as np
# Enter your code here. Read input from STDIN. Print output to STDOUT
def ndarray(array_input):
#Write your code here
x1=np.array(array_input)
print(x1.ndim)
print(x1.shape)
print(x1.size)
Lab 4: Try it Out - ndarray with Shapes [Welcome to Python - ndarray shape - Hands-on]
Try it Out - Python "ndarray shape" Hands-on
Solution: Python "ndarray shape" Hands-on
# ndarray shape
import numpy as np
# Enter your code here. Read input from STDIN. Print output to STDOUT
def ndshape(d, shape1):
#Write your code here
x = np.eye(d)
print(x)
print(np.ones(shape1))
if __name__ == "__main__":
d=int(input())
shape1=list(map(int,input().split()))
ndshape(d, shape1)
LAB 5. Try it Out - 3D array [Welcome to Python - 3D Array - Hands-on]
LAB 5. Python - 3D Array
Solution: 3D array
import numpy as np
# Enter your code here. Read input from STDIN. Print output to STDOUT
def array_3d(n, x, y, z):
#Write your code below
np.random.seed(100)
x1 = 5 + 2.5*np.random.randn(n) # normal distribution with mean=5 and sd=2.5
A=x1.nbytes
m = np.random.uniform(0,1,x*y*z)
x2 = m.reshape(x,y,z)
print(A)
print(x2)
if __name__ == "__main__":
n=int(input())
x, y, z=list(map(int,input().split()))
array_3d(n, x, y, z)
Lab 6 : Try it Out - More on ndarray [Welcome to Python - More on ndarray - Hands-on]
Lab 6 : More on ndarray
Solution: More on ndarray
import numpy as np
# Enter your code here. Read input from STDIN. Print output to STDOUT
def arr(a, x, y, z):
#Write your code here
x1=np.arange(2,a,2)
x2=np.linspace(x, y, z)
print(x1)
print(x2)
if __name__ == "__main__":
a=int(input())
x, y, z=list(map(int,input().split()))
arr(a, x, y, z)
LAB 7 : Try it Out - Array Manipulation 1 [Welcome to Python - Array Manipulation 1 - Hands-on]
LAB 7: Python - Array Manipulation 1 - Hands-on
Solution: Array Manipulation 1 - Hands-on
import numpy as np
# Enter your code here. Read input from STDIN. Print output to STDOUT
def array_split(i,r,c):
#Write your code below
x=np.arange(i)
y = np.array(x).reshape(r,c)
a,b = np.hsplit(y,2)
print(a)
print(b)
if __name__== "__main__":
i=int(input())
r,c = list(map(int,input().split()))
array_split(i,r,c)
Lab 8 : : Try it Out - Array Manipulation 2 [Welcome to Array Manipulation2]
Lab 8 : : Array Manipulation 2 - Hands-on
Solution: Array Manipulation 2 - Hands-on
import ast
import numpy as np
# Enter your code here. Read input from STDIN. Print output to STDOUT
def array_split(n,n_row,n_col):
x =np.arange(n)
z= np.array(x, ).reshape(n_row,n_col)
a,b=np.vsplit(z,2)
print(a)
print(b)
if __name__ == "__main__":
n = ast.literal_eval(input())
n_row = ast.literal_eval(input())
n_col = ast.literal_eval(input())
array_split(n,n_row,n_col)
Lab 9 : : Try it Out - Join Arrays [Welcome to Join Arrays]
Lab 9 : : Welcome to Join Arrays - Hands-on
Solution: Welcome to Join Arrays - Hands-on
import numpy as np
import ast
# Enter your code here. Read input from STDIN. Print output to STDOUT
def join_array(list1,list2):
p= np.array(list1).reshape(2,2)
q= np.array(list2).reshape(2,3)
print(np.hstack((p,q)))
if __name__ == "__main__":
l1 = ast.literal_eval(input())
l2 = ast.literal_eval(input())
join_array(l1,l2)
LAB 10 : : Welcome to Operations on Arrays 1 - Hands-on
LAB 10 : : Welcome to Operations on Arrays 1 - Hands-on
Solution: Welcome to Operations on Arrays 1
import numpy as np
# Enter your code here. Read input from STDIN. Print output to STDOUT
def array_oper(num1,num2):
y= np.arange(num1,num2+1)
y=y.reshape(2,3)
y=y**2
y=y+5
print(y)
if __name__ == "__main__":
num1 = int(input())
num2 = int(input())
array_oper(num1,num2)
LAB 11: Welcome to Operation on Arrays 2 - Hands-on
LAB 11: Welcome to Operation on Arrays 2 - Hands-on
Solution: Welcome to Operation on Arrays 2 - Hands-on
import numpy as np
#Enter your code here. Read input from STDIN. Print output to STDOUT
def array_oper(num1,num2):
np.random.seed(100)
r=np.random.randint(num1,num2, 30).reshape(5,6)
x= np.sum(r,axis=0)
y=np.sum(r,axis=1)
print(x[-1])
print(y[-1])
if __name__ == "__main__":
num1 = int(input())
num2 = int(input())
array_oper(num1,num2)
LAB 12: Welcome to Operation on Arrays 3 - Hands-on
LAB 12 : : Welcome to Operation on Arrays 3 - Hands-on
Solution: Welcome to Operation on Arrays 3
import numpy as np
import ast
# Enter your code here. Read input from STDIN. Print output to STDOUT
def array_oper(n,m,sd):
np.random.seed(100)
x=m + sd*np.random.randn(n)
print(np.mean(x))
print(np.std(x))
print(np.var(x))
if __name__ == '__main__':
num = ast.literal_eval(input())
mean = ast.literal_eval(input())
sd = ast.literal_eval(input())
array_oper(num,mean,sd)
LAB 13: : Try it Out - Array Indexing [Welcome to Array Indexing]
LAB 13: : Welcome to Array Indexing - Hands-on
Solution: Welcome to Array Indexing
import numpy as np
# Enter your code here. Read input from STDIN. Print output to STDOUT
def array_index(n,n_row,n_col):
x= np.arange(n).reshape(n_row,n_col)
print(x[-1])
print(x[:,2])
print(x[ :2, 2:])
if __name__ == '__main__':
n = int(input())
n_row = int(input())
n_col = int(input())
array_index(n,n_row,n_col)
LAB 14: Try it Out - Slicing [Welcome to Slicing]
LAB 14: Welcome to Slicing - Hands-on
Solution: Welcome to Slicing
import numpy as np
# Enter your code here. Read input from STDIN. Print output to STDOUT
def array_slice(n,n_dim,n_row,n_col):
x= np.arange(n).reshape(n_dim,n_row,n_col)
b=np.array([True, False])
print(x[b])
print(x[b,:,1:3])
if __name__ == '__main__':
n = int(input())
n_dim = int(input())
n_row = int(input())
n_col = int(input())
array_slice(n,n_dim,n_row,n_col)