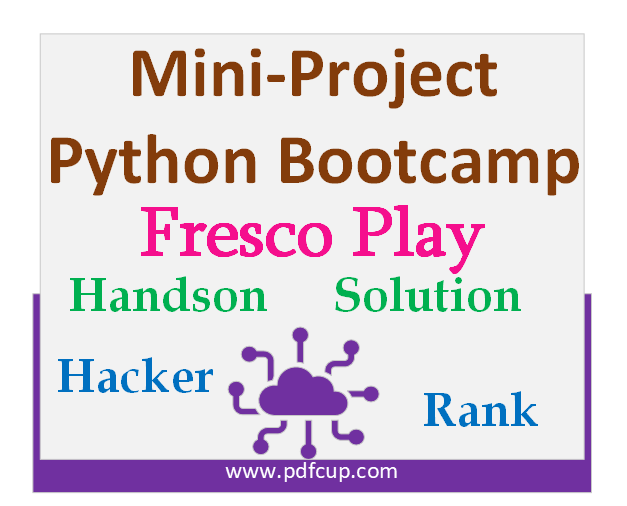
Kindly Note: If you had any problems, please comment below.
LAB 1 Welcome to Python Lists
LAB 1 : : Welcome to Python Lists
#!/bin/python3
import os
import sys
import math
import random
import re
#
#
class IncreasingList:
temp_list=[]
def append(self, val):
lst= IncreasingList.temp_list
"""
first, it removes all elements from the list that have greater values than val, starting from the last one, and once there are no greater element in the list, it appends val to the end of the list
"""
run=True
while run:
if len(lst)<1:
lst.append (val)
break
elif max(lst)>val:
indx=lst.index(max (lst))
lst.pop(indx)
else :
lst.append (val)
run=False
IncreasingList.temp_list = lst
def pop(self):
"""
removes the last element from the list if the list is not empty, otherwise, if the list is empty, it does nothing
"""
if len (IncreasingList.temp_list)>0:
IncreasingList.temp_list.pop()
def __len__(self):
"""
returns the number of elements in the list
"""
return len(IncreasingList.temp_list)
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == '__main__' :
fptr = open (os.environ['OUTPUT_PATH' ], 'w' )
lst = IncreasingList ()
q = int(input ())
for _ in range(q):
op = input().split()
op_name = op[0]
if op_name == "append" :
val = int(op[1])
lst.append(val)
elif op_name == "pop" :
lst.pop()
elif op_name == "size" :
fptr.write("%d\n" % len(lst))
else :
raise ValueError ("invalid operation")
fptr.close()
LAB 2 Welcome to Python Files
Question 1: Python Files 1
Solution : Python Files 1
#!/bin/python3
import os
import sys
#
#
def multiStr():
zenPython = '''The Zen of Python, by Tim Peters
Beautiful is better than ugly.
Explicit is better than implicit.
Simple is better than complex.
Complex is better than complicated.
Flat is better than nested.
Sparse is better than dense.
Readability counts.
Special cases aren't special enough to break the rules.
Although practicality beats purity.
Errors should never pass silently.
Unless explicitly silenced.
In the face of ambiguity, refuse the temptation to guess.
There should be one-- and preferably only one --obvious way to do it.
Although that way may not be obvious at first unless you're Dutch.
Now is better than never.
Although never is often better than *right* now.
If the implementation is hard to explain, it's a bad idea.
If the implementation is easy to explain, it may be a good idea.
Namespaces are one honking great idea -- let's do more of those!
'''
return (zenPython)
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == "__main__":
f = open(os.environ['OUTPUT_PATH'], 'w')
res = multiStr();
f.write(res + "\n")
f.close()
Question 2: Python Files 2
Solution : Python Files 2
#!/bin/python3
import os
import sys
import io
#
#
def main():
zenPython = '''The Zen of Python, by Tim Peters
Beautiful is better than ugly.
Explicit is better than implicit.
Simple is better than complex.
Complex is better than complicated.
Flat is better than nested.
Sparse is better than dense.
Readability counts.
Special cases aren't special enough to break the rules.
Although practicality beats purity.
Errors should never pass silently.
Unless explicitly silenced.
In the face of ambiguity, refuse the temptation to guess.
There should be one-- and preferably only one --obvious way to do it.
Although that way may not be obvious at first unless you're Dutch.
Now is better than never.
Although never is often better than *right* now.
If the implementation is hard to explain, it's a bad idea.
If the implementation is easy to explain, it may be a good idea.
Namespaces are one honking great idea -- let's do more of those!
'''
#Create fp implementation, check for fp instance and return status
fp = io.StringIO(zenPython)
return fp
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == "__main__":
f = open(os.environ['OUTPUT_PATH'], 'w')
res = main();
f.write(str('io.StringIO' in str(res)) + "\n")
f.close()
Question 3: Python Files 3
Solution : Python Files 3
#!/bin/python3
import os
import sys
import io
#
# Complete the function below.
def main():
zenPython = '''
The Zen of Python, by Tim Peters
Beautiful is better than ugly.
Explicit is better than implicit.
Simple is better than complex.
Complex is better than complicated.
Flat is better than nested.
Sparse is better than dense.
Readability counts.
Special cases aren't special enough to break the rules.
Although practicality beats purity.
Errors should never pass silently.
Unless explicitly silenced.
In the face of ambiguity, refuse the temptation to guess.
There should be one-- and preferably only one --obvious way to do it.
Although that way may not be obvious at first unless you're Dutch.
Now is better than never.
Although never is often better than *right* now.
If the implementation is hard to explain, it's a bad idea.
If the implementation is easy to explain, it may be a good idea.
Namespaces are one honking great idea -- let's do more of those!
'''
fp = io.StringIO(zenPython)
#Add Implementation step here
zenlines = fp.readlines()
return zenlines[0:5]
'''For testing the code, no input is to be provided'''
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == "__main__":
f = open(os.environ['OUTPUT_PATH'], 'w')
res = main();
f.write(str(res) + "\n")
f.close()
Question 4: Python Files 4
Solution : Python Files 4
#!/bin/python3
import os
import sys
import io
#
# Complete the function below.
def main():
zenPython = '''
The Zen of Python, by Tim Peters
Beautiful is better than ugly.
Explicit is better than implicit.
Simple is better than complex.
Complex is better than complicated.
Flat is better than nested.
Sparse is better than dense.
Readability counts.
Special cases aren't special enough to break the rules.
Although practicality beats purity.
Errors should never pass silently.
Unless explicitly silenced.
In the face of ambiguity, refuse the temptation to guess.
There should be one-- and preferably only one --obvious way to do it.
Although that way may not be obvious at first unless you're Dutch.
Now is better than never.
Although never is often better than *right* now.
If the implementation is hard to explain, it's a bad idea.
If the implementation is easy to explain, it may be a good idea.
Namespaces are one honking great idea -- let's do more of those!
'''
fp = io.StringIO(zenPython)
zenlines = fp.readlines()
#Add implementation step here
zenlines = [ line.strip() for line in zenlines ]
return zenlines
'''For testing the code, no input is to be provided'''
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == "__main__":
f = open(os.environ['OUTPUT_PATH'], 'w')
res = main();
f.write(str(res) + "\n")
f.close()
Question 5: Python Files 5
Solution: Python Files 5
#!/bin/python3
import os
import sys
import io
import re
#
# Complete the function below.
def main():
zenPython = '''
The Zen of Python, by Tim Peters
Beautiful is better than ugly.
Explicit is better than implicit.
Simple is better than complex.
Complex is better than complicated.
Flat is better than nested.
Sparse is better than dense.
Readability counts.
Special cases aren't special enough to break the rules.
Although practicality beats purity.
Errors should never pass silently.
Unless explicitly silenced.
In the face of ambiguity, refuse the temptation to guess.
There should be one-- and preferably only one --obvious way to do it.
Although that way may not be obvious at first unless you're Dutch.
Now is better than never.
Although never is often better than *right* now.
If the implementation is hard to explain, it's a bad idea.
If the implementation is easy to explain, it may be a good idea.
Namespaces are one honking great idea -- let's do more of those!
'''
fp = io.StringIO(zenPython)
zenlines = fp.readlines()
zenlines = [ line.strip() for line in zenlines ]
#Add implementation here to set and match patterns
#Add portions implementation here
portions=re.findall(r"[-*] ?([^-*].*?) ?[-*]",zenPython)
return portions
'''For testing the code, no input is to be provided'''
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == "__main__":
f = open(os.environ['OUTPUT_PATH'], 'w')
res = main();
f.write(str(res) + "\n")
f.close()
LAB 3 Welcome to Python - Class and Static Methods
Question 1: Class and Static Mathods 1
Question 1: Class and Static Mathods 1
#!/bin/python3
import os
import sys
#Add Circle class implementation below
class Circle():
no_of_circles =0
def __init__(self,x):
self.radius=x
self.no_of_circles =0
def area(self):
Area= 3.14*(self.radius**2)
Circle.no_of_circles+=1
return Area
'''Check the Tail section for input/output'''
# if __name__ == '__main__':
# Starting from here:
if __name__ == "__main__":
with open(os.environ['OUTPUT_PATH'], 'w') as fout:
res_lst = list()
lst = list(map(lambda x: float(x.strip()), input().split(',')))
for radius in lst:
res_lst.append(Circle(radius).area())
fout.write("{}\n{}".format(str(res_lst), str(Circle.no_of_circles)))
Question 2: Class and Static Mathods 2
/Question 2: Class and Static Mathods 2
#!/bin/python3
import os
import sys
#Add Circle class implementation here
class Circle:
no_of_circles =0
def __init__(self,v) :
self.radius=v
def area(self):
Area= 3.14*(self.radius**2)
#Circle.no_of_circles+=1
return Area
@classmethod
def getCircleCount(self):
Circle.no_of_circles+=1
return Circle.no_of_circles
# Note: One more change need to be done in last line of "__main__" section before submitting the code.
'''Check the Tail section for input/output'''
# if __name__ == '__main__':
# Starting from here:
if __name__ == "__main__":
with open(os.environ['OUTPUT_PATH'], 'w') as fout:
res_lst = list()
circcount = list()
lst = list(map(lambda x: float(x.strip()), input().split(',')))
for radi in lst:
c=Circle(radi)
res_lst.append(str(c.getCircleCount())+" : "+str(c.area()))
fout.write("{}\n{}".format(str(res_lst), str(Circle.getCircleCount()-1)))
Question 3: Class and Static Mathods 3
Question 3: Class and Static Mathods 3
#!/bin/python3
import os
import sys
#Add circle class implementation here
class Circle:
no_of_circles =0
def __init__(self,v) :
self.radius=v
def area(self):
Area= 3.14*(self.radius**2)
#Circle.no_of_circles+=1
return Area
@classmethod
def getCircleCount(self):
Circle.no_of_circles+=1
return Circle.no_of_circles
'''Check the Tail section for input/output'''
# if __name__ == '__main__':
# Starting from here:
if __name__ == "__main__":
with open(os.environ['OUTPUT_PATH'], 'w') as fout:
res_lst = list()
circcount = list()
lst = list(map(lambda x: float(x.strip()), input().split(',')))
for radi in lst:
c=Circle(radi)
res_lst.append(str(c.getCircleCount())+" : "+str(c.area()))
fout.write("{}".format(str(res_lst)))
LAB 4 Welcome to Python Descriptors
LAB 4 Welcome to Python - Descriptors
#!/bin/python3
import sys
import os
# Add Celsius class implementation below.
class Celsius:
def __get__(self , object_of_Temperature , owner_Temperature ):
return 5 * (object_of_Temperature.fahrenheit - 32) / 9
def __set__(self, object_of_Temperature, celsius):
object_of_Temperature.fahrenheit = 32 + 9 * celsius / 5
# Add temperature class implementation below.
class Temperature:
celsius = Celsius()
def __init__(self, user_fahrenheit):
self.fahrenheit = user_fahrenheit
'''Check the Tail section for input/output'''
# if __name__ == '__main__':
# Starting from here:
if __name__ == "__main__":
with open(os.environ['OUTPUT_PATH'], 'w') as fout:
res_lst = list()
t1 = Temperature(int(input()))
res_lst.append((t1.fahrenheit, t1.celsius))
t1.celsius = int(input())
res_lst.append((t1.fahrenheit, t1.celsius))
fout.write("{}\n{}".format(*res_lst))
LAB 5 Welcome to Python - Python Decorators
Question 1: Decorators 1
Solution 1: Decorators-1
#!/bin/python3
import sys
import os
import datetime as dt
#Add log function and inner function implementation here
def log(func):
def inner(*args, **kwdargs):
STDOUT= "Accessed the function -'{}' with arguments {} {}".format(func.__name__,args,kwdargs)
return STDOUT
return inner
def greet(msg):
'Greeting Message : ' + msg
greet = log(greet)
'''Check the Tail section for input/output'''
# if __name__ == '__main__':
# Starting from here:
if __name__ == "__main__":
with open(os.environ['OUTPUT_PATH'], 'w') as fout:
res_lst = list()
res_lst.append(greet(str(input())))
fout.write("{}".format(*res_lst))
Question 2: Decorators 2
Solution 2: Decorators-2
#!/bin/python3
import sys
import os
def log(func):
def inner(*args, **kwdargs):
str_template = "Accessed the function -'{}' with arguments {} {}".format(func.__name__, args, kwdargs)
return str_template + "\n" + str(func(*args, **kwdargs))
return inner
#Add greet function definition here
def greet(msg):
'Greeting Message : ' + msg
@log
def average(n1,n2,n3):
return (n1+n2+n3)/3
greet = log(greet)
'''Check the Tail section for input/output'''
# if __name__ == '__main__':
# Starting from here:
if __name__ == "__main__":
with open(os.environ['OUTPUT_PATH'], 'w') as fout:
res_lst = list()
(a,b,c) = (map(lambda x: float(x.strip()), input().split(',')))
res_lst.append(average(a,b,c))
fout.write("{}".format(*res_lst))
Question 3: Decorators 3
Solution 3: Decorators-3
#!/bin/python3
import sys
import os
#Define and implement bold_tag
def bold_tag(func):
def inner(*args, **kwdargs):
return "" +str(func(*args, **kwdargs))+""
return inner
@bold_tag
def say(msg):
return msg
'''Check the Tail section for input/output'''
# if __name__ == '__main__':
# Starting from here:
if __name__ == "__main__":
with open(os.environ['OUTPUT_PATH'], 'w') as fout:
res_lst = list()
res_lst.append(say(input()))
fout.write("{}".format(*res_lst))
Question 4: Decorators 4
Solution 4: Decorators-4
#!/bin/python3
import os
import sys
def bold_tag(func):
def inner(*args, **kwdargs):
return ''+func(*args, **kwdargs)+''
return inner
#Implement italic_tag below
def italic_tag(func):
def inner(*args, **kwdargs):
return '' + func(*args, **kwdargs) + ''
return inner
@italic_tag
def say(msg):
return msg
'''Check the Tail section for input/output'''
# if __name__ == '__main__':
# Starting from here:
if __name__ == "__main__":
with open(os.environ['OUTPUT_PATH'], 'w') as fout:
res_lst = list()
res_lst.append(say(input()))
fout.write("{}".format(*res_lst))
Question 5: Decorators 5
Solution 5: Decorators-5
#!/bin/python3
import os
import sys
def bold_tag(func):
def inner(*args, **kwdargs):
return ''+func(*args, **kwdargs)+''
return inner
def italic_tag(func):
def inner(*args, **kwdargs):
return '' + func(*args, **kwdargs) + ''
return inner
#Add greet() function definition
@italic_tag
def greet():
user_input= input()
return user_input
'''Check the Tail section for input/output'''
# if __name__ == '__main__':
# Starting from here:
if __name__ == "__main__":
with open(os.environ['OUTPUT_PATH'], 'w') as fout:
res_lst = list()
res_lst.append(greet())
fout.write("{}".format(*res_lst))
Question 6: Decorators 6
Solution 6: Decorators-6
#!/bin/python3
import os
import sys
def bold_tag(func):
def inner(*args, **kwdargs):
return ''+func(*args, **kwdargs)+''
return inner
def italic_tag(func):
def inner(*args, **kwdargs):
return '' + func(*args, **kwdargs) + ''
return inner
#Add greet() implementation here
@italic_tag
@bold_tag
def greet():
user_input= input()
return user_input
'''check Tail section below for input / output'''
# if __name__ == '__main__':
# Starting from here:
if __name__ == "__main__":
with open(os.environ['OUTPUT_PATH'], 'w') as fout:
res_lst = list()
res_lst.append(greet())
fout.write("{}".format(*res_lst))
Toipc Covered:
Mini-Project Python Bootcamp: Fresco Play Solution - Hacker Rank
Mini-Project - Python 3 Programming
About the author

I'm a professor at National University's Department of Computer Science. My main streams are data science and data analysis. Project management for many computer science-related sectors. Next working project on Al with deep Learning.....