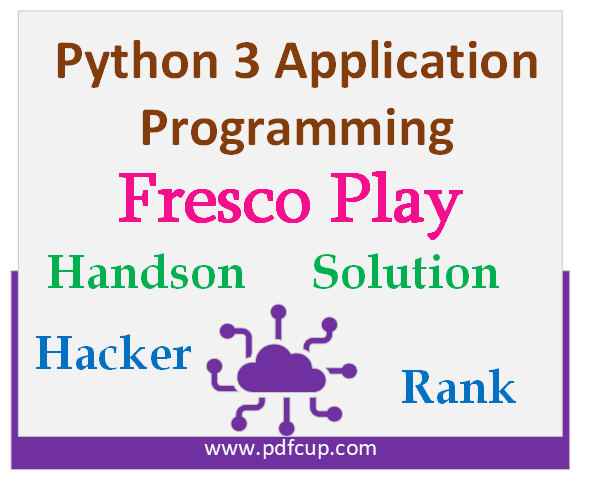
Kindly Note: If you had any problem in code execution, please comment below.
Also Read, Python3 - Functions and OOPs Fresco Play Hands-on Solution..
LAB 1: Python Decorators - Hands-on
Question 1: Python Decorators 1- Hands-on
Solution 1: Python Decorators 1
#!/bin/python3
import sys
import os
import datetime as dt
#Add log function and inner function implementation here
def log(func):
def inner(*args, **kwdargs):
STDOUT= "Accessed the function -'{}' with arguments {} {}".format(func.__name__,args,kwdargs)
return STDOUT
return inner
def greet(msg):
'Greeting Message : ' + msg
greet = log(greet)
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == "__main__":
with open(os.environ['OUTPUT_PATH'], 'w') as fout:
res_lst = list()
res_lst.append(greet(str(input())))
fout.write("{}".format(*res_lst))
Question 2: Python Decorator 2
Solution 2: Python Decorator 2
#!/bin/python3
import sys
import os
def log(func):
def inner(*args, **kwdargs):
str_template = "Accessed the function -'{}' with arguments {} {}".format(func.__name__,args,kwdargs)
return str_template + "\n" + str(func(*args, **kwdargs))
return inner
#Add greet function definition here
@log
def average(n1,n2,n3):
return (n1+n2+n3)/3
'''Check the Tail section for input/output'''
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == "__main__":
with open(os.environ['OUTPUT_PATH'], 'w') as fout:
res_lst = list()
(a,b,c) = (map(lambda x: float(x.strip()), input().split(',')))
res_lst.append(average(a,b,c))
fout.write("{}".format(*res_lst))
Question 3: Python Decorator 3
Solution 3: Python Decorator 3
#!/bin/python3
import sys
import os
#Define and implement bold_tag
def bold_tag(func):
def inner(*args, **kwdargs):
return '<b>'+func(*args, **kwdargs)+'</b>'
return inner
def say(msg):
return msg
say=bold_tag(say)
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == "__main__":
with open(os.environ['OUTPUT_PATH'], 'w') as fout:
res_lst = list()
res_lst.append(say(input()))
fout.write("{}".format(*res_lst))
Question 4: Python Decorator 4
Solution 4: Python Decorator 4
#!/bin/python3
import os
import sys
def bold_tag(func):
def inner(*args, **kwdargs):
return ''+func(*args, **kwdargs)+''
return inner
#Implement italic_tag below
def italic_tag(func):
def inner(*args, **kwdargs):
return '' + func(*args, **kwdargs) +''
return inner
def say(msg):
return msg
say=italic_tag(say)
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == "__main__":
with open(os.environ['OUTPUT_PATH'], 'w') as fout:
res_lst = list()
res_lst.append(say(input()))
fout.write("{}".format(*res_lst))
Question 5: Python Decorator 5
Solution 5: Python Decorator 5
#!/bin/python3
import os
import sys
def bold_tag(func):
def inner(*args, **kwdargs):
return ''+func(*args, **kwdargs)+''
return inner
def italic_tag(func):
def inner(*args, **kwdargs):
return '' + func(*args, **kwdargs) + ''
return inner
#Add greet() function definition
@italic_tag
def greet():
msg=input()
return msg
'''Check the Tail section for input/output'''
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == "__main__":
with open(os.environ['OUTPUT_PATH'], 'w') as fout:
res_lst = list()
res_lst.append(greet())
fout.write("{}".format(*res_lst))
Question 6: Python Decorator 6
Solution 6: Python Decorator 6
#!/bin/python3
import os
import sys
def bold_tag(func):
def inner(*args, **kwdargs):
return ''+func(*args, **kwdargs)+''
return inner
def italic_tag(func):
def inner(*args, **kwdargs):
return '' + func(*args, **kwdargs) + ''
return inner
#Add greet() implementation here
@italic_tag
@bold_tag
def greet():
msg=input()
return msg
'''check Tail section below for input / output'''
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == "__main__":
with open(os.environ['OUTPUT_PATH'], 'w') as fout:
res_lst = list()
res_lst.append(greet())
fout.write("{}".format(*res_lst))
LAB 2 : : Welcome to Python - Class and Static Methods
Question 1: Class and Static Methods 1
Solution 1: Class and Static Methods 1
#!/bin/python3
import os
import sys
#Add Circle class implementation below
class Circle():
no_of_circles =0
def __init__(self,x) :
self.radius=x
self.no_of_circles =0
def area(self):
Area= 3.14*(self.radius**2)
Circle.no_of_circles+=1
return Area
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == "__main__":
with open(os.environ['OUTPUT_PATH'], 'w') as fout:
res_lst = list()
lst = list(map(lambda x: float(x.strip()), input().split(',')))
for radius in lst:
res_lst.append(Circle(radius).area())
fout.write("{}\n{}".format(str(res_lst), str(Circle.no_of_circles)))
Question 2: Class and Static Methods 2
Solution 2: Class and Static Methods 2
#!/bin/python3
import os
import sys
#Add Circle class implementation here
class Circle:
no_of_circles =0
def __init__(self,v) :
self.radius=v
def area(self):
Area= 3.14*(self.radius**2)
#Circle.no_of_circles+=1
return Area
@classmethod
def getCircleCount(self):
Circle.no_of_circles+=1
return Circle.no_of_circles
# Refer '__main__' method code which is given below if required.
# Kindly note: One change need to do in '__main__' code, check the last line value '-1' need to add there.
# if __name__ == '__main__':
# Starting from here:
if __name__ == "__main__":
with open(os.environ['OUTPUT_PATH'], 'w') as fout:
res_lst = list()
circcount = list()
lst = list(map(lambda x: float(x.strip()), input().split(',')))
for radi in lst:
c=Circle(radi)
res_lst.append(str(c.getCircleCount())+" : "+str(c.area()))
fout.write("{}\n{}".format(str(res_lst), str(Circle.getCircleCount()-1)))
Question 3: Class and Static Methods 3
Solution 3: Class and Static Methods 3
#!/bin/python3
import os
import sys
class Circle:
no_of_circles =0
def __init__(self,v) :
self.radius=v
def area(self):
Area= 3.14*(self.radius**2)
return Area
@classmethod
def getCircleCount(self):
Circle.no_of_circles+=1
return Circle.no_of_circles
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == "__main__":
with open(os.environ['OUTPUT_PATH'], 'w') as fout:
res_lst = list()
circcount = list()
lst = list(map(lambda x: float(x.strip()), input().split(',')))
for radi in lst:
c=Circle(radi)
res_lst.append(str(c.getCircleCount())+" : "+str(c.area()))
fout.write("{}".format(str(res_lst)))
LAB 3: Welcome to Python - Higher order functions and closures 1
Question 1: Welcome to Python - Higher order functions and closures - 1
Solution 1: Welcome to Python - Higher order functions and closures '1'
#!/bin/python3
import sys
import os
#Write detecter implementation
def detecter(x):
search_element= x
#Write isIn implementation
def isIn (lst):
user_input_list= lst
if search_element in user_input_list:
return "True"
else:
return "False"
return isIn
detect30=detecter(30)
detect45=detecter(45)
#Write closure function implementation for detect30 and detect45
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == "__main__":
with open(os.environ['OUTPUT_PATH'], 'w') as fout:
func_lst = [detect30, detect45]
res_lst = list()
lst = list(map(lambda x: int(x.strip()), input().split(',')))
for func in func_lst:
res_lst.append(func(lst))
fout.write("{}\n{}".format(*res_lst))
Question 2: Welcome to Python - Higher order functions and closures - 2
Solution 2: Welcome to Python - Higher order functions and closures '2'
#!/bin/python3
import sys
import os
# Add the factory function implementation here
def factory(x=0):
def current():
return x
def counter():
return x+1
return current,counter
f_current, f_counter = factory(int(input()))
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == "__main__":
with open(os.environ['OUTPUT_PATH'], 'w') as fout:
func_lst = [f_current, f_counter]
res_lst = list()
for func in func_lst:
res_lst.append(func())
fout.write("{}\n{}".format(*res_lst))
LAB 4: Defining an Abstract Class in Python
Defining an Abstract Class in Python:
Solution:-
#!/bin/python3
import inspect
from abc import ABC, abstractmethod
# Define the abstract class 'Animal' below
# with abstract method 'say'
class Animal(ABC):
@abstractmethod
def say(self):
pass
# Define class Dog derived from Animal
# Also define 'say' method inside 'Dog' class
class Dog(Animal):
def say(self):
return "I speak Booooo"
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == '__main__':
if issubclass(Animal, ABC):
print("'Animal' is an abstract class" )
if '@abstractmethod' in inspect.getsource(Animal.say):
print("'say' is an abstract method")
if issubclass(Dog, Animal):
print("'Dog' is dervied from 'Animal' class" )
d1 = Dog()
print("Dog,'d1', says :", d1.say())
LAB 5: Context Managers
Question 1: Writing text to a file using "with".
Solution 1:-
#!/bin/python3
#!/bin/python3
import sys
import os
import inspect
# Complete the function below.
def writeTo(filename, input_text):
with open(filename, mode="w") as my_file:
return my_file.writelines(input_text)
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == "__main__":
try:
filename = str(input())
except:
filename = None
try:
input_text = str(input())
except:
input_text = None
res = writeTo(filename, input_text)
if 'with' in inspect.getsource(writeTo):
print("'with' used in 'writeTo' function definition.")
if os.path.exists(filename):
print('File :',filename, 'is present on system.')
with open(filename) as fp:
content = fp.read()
if content == input_text:
print('File Contents are :', content)
Question 2: Archiving file using 'with'.
Solution 2:-
#!/bin/python3
import zipfile
import sys
import os
import inspect
# Define 'writeTo' function below, such that
# it writes input_text string to filename.
def writeTo(filename, input_text):
with open(filename, mode="w") as my_file:
return my_file.writelines(input_text)
# Define the function 'archive' below, such that
# it archives 'filename' into the 'zipfile'
def archive(zfile, filename):
with zipfile.ZipFile(zfile, mode="w") as z:
return z
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == "__main__":
try:
filename = str(input())
except:
filename = None
try:
input_text = str(input())
except:
input_text = None
try:
zip_file = str(input())
except:
zip_file = None
res = writeTo(filename, input_text)
if 'with' in inspect.getsource(writeTo):
print("'with' used in 'writeTo' function definition.")
if os.path.exists(filename):
print('File :',filename, 'is present on system.')
res = archive(zip_file, filename)
if 'with' in inspect.getsource(archive):
print("'with' used in 'archive' function definition.")
if os.path.exists(zip_file):
print('ZipFile :',zip_file, 'is present on system.')
Question 3: Run a System Command using Popen utility of Subprocess module.
Solution 3:-
#!/bin/python3
import sys
import os
import subprocess
import inspect
# Complete the function below.
def run_process(cmd_args):
with subprocess.Popen(cmd_args, stdout= subprocess.PIPE)as w:
return w.communicate()[0]
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == "__main__":
f = open(os.environ['OUTPUT_PATH'], 'w')
cmd_args_cnt = 0
cmd_args_cnt = int(input())
cmd_args_i = 0
cmd_args = []
while cmd_args_i < cmd_args_cnt:
try:
cmd_args_item = str(input())
except:
cmd_args_item = None
cmd_args.append(cmd_args_item)
cmd_args_i += 1
res = run_process(cmd_args);
#f.write(res.decode("utf-8") + "\n")
if 'with' in inspect.getsource(run_process):
f.write("'with' used in 'run_process' function definition.\n")
if 'Popen' in inspect.getsource(run_process):
f.write("'Popen' used in 'run_process' function definition.\n")
f.write('Process Output : %s\n' % (res.decode("utf-8")))
f.close()
LAB 6: Welcome to Python - Coroutines New
Question 1: Welcome to Python - Coroutines New.
Solution 1:-
#!/bin/python3
import sys
# Define the coroutine function 'linear_equation' below.
def linear_equation(a, b):
while True:
x= yield
expression= a*(x**2)+b
print("Expression, {}*x^2 + {}, with x being {} equals {}".format(a,b,x,expression))
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == "__main__":
a = float(input())
b = float(input())
equation1 = linear_equation(a, b)
next(equation1)
equation1.send(6)
Question 2: Define a decorator for coroutine.
Solution 2:-
#!/bin/python3
import sys
import os
# Define 'coroutine_decorator' below
def coroutine_decorator(coroutine_func):
#def start(*args, **kwargs):
def next_iter(*args):
#cr = coroutine_func(*args, **kwargs)
cr=coroutine_func(*args)
next(cr)
return cr
return next_iter
# Define coroutine 'linear_equation' as specified in previous exercise
@coroutine_decorator
def linear_equation(a, b):
while True:
x= yield
expression= a*(x**2)+b
print("Expression, {}*x^2 + {}, with x being {} equals {}".format(a,b,x,expression))
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == "__main__":
a = float(input())
b = float(input())
equation1 = linear_equation(a, b)
equation1.send(6)
Question 3: Linking Two coroutines.
Solution 3:-
#!/bin/python3
import sys
# Define the function 'coroutine_decorator' below
def coroutine_decorator(coroutine_func):
#def start(*args, **kwargs):
def next_iter(*args):
#cr = coroutine_func(*args, **kwargs)
cr=coroutine_func(*args)
next(cr)
return cr
return next_iter
# Define the coroutine function 'linear_equation' below
@coroutine_decorator
def linear_equation(a, b):
while True:
x= yield
expression= a*(x**2)+b
print("Expression, {}*x^2 + {}, with x being {} equals {}".format(a,b,x,expression))
# Define the coroutine function 'numberParser' below
@coroutine_decorator
def numberParser():
equation1 = linear_equation(3, 4)
equation2 = linear_equation(2, -1)
# code to send the input number to both the linear equations
while True:
x= yield
equation1.send(x)
equation2.send(x)
def main(x):
n = numberParser()
n.send(x)
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == "__main__":
x = float(input())
res = main(x);
LAB 7: Give a Try - Database Connectivity : : Welcome to Python - Database Connectivity.
Question 1: Database Connectivity 1- Connect to Database and create Table.
Solution 1:-
#!/bin/python3
import sys
import os
import sqlite3
# Complete the following function:
def main():
SQL_Connection = sqlite3.connect('SAMPLE.db')
#create connection cursor
cur= SQL_Connection.cursor()
#create table ITEMS using the cursor
cur.execute("drop table if exists ITEMS")
sql_query="create table ITEMS (item_id, item_name, item_description, item_category, quantity_in_stock)"
cur.execute(sql_query)
#commit connection
SQL_Connection.commit()
#close connection
SQL_Connection.close()
'''To test the code, no input is required'''
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == "__main__":
f = open(os.environ['OUTPUT_PATH'], 'w')
res = main();
f.write(str(res) + "\n")
f.close()
Question 2: Database Connectivity 2 - Insert records in table.
Solution 2:-
#!/bin/python3
import sys
import os
import sqlite3
# Complete the function below.
def main():
SQL_Connection = sqlite3.connect('SAMPLE.db')
cursor = SQL_Connection.cursor()
cursor.execute("drop table if exists ITEMS")
sql_statement = '''CREATE TABLE ITEMS
(item_id integer not null, item_name varchar(300),
item_description text, item_category text,
quantity_in_stock integer)'''
cursor.execute(sql_statement)
items = [(101, 'Nik D300', 'Nik D300', 'DSLR Camera', 3),
(102, 'Can 1300', 'Can 1300', 'DSLR Camera', 5),
(103, 'gPhone 13S', 'gPhone 13S', 'Mobile', 10),
(104, 'Mic canvas', 'Mic canvas', 'Tab', 5),
(105, 'SnDisk 10T', 'SnDisk 10T', 'Hard Drive', 1)
]
#Add code to insert records to ITEM table
try:
sql= """insert into ITEMS VALUES(?,?,?,?,?)"""
cursor.executemany(sql,items)
cursor.execute("select * from ITEMS")
except:
return 'Unable to perform the transaction.'
rowout=[]
for row in cursor.fetchall():
rowout.append(row)
return rowout
SQL_Connection.close()
'''For testing the code, no input is required'''
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == "__main__":
f = open(os.environ['OUTPUT_PATH'], 'w')
res = main();
f.write(str(res) + "\n")
f.close()
Question 3: Database Connectivity 3 - Select records from table.
Solution 3:-
#!/bin/python3
import sys
import os
import sqlite3
# Complete the function below.
def main():
conn = sqlite3.connect('SAMPLE.db')
cursor = conn.cursor()
cursor.execute("drop table if exists ITEMS")
sql_statement = '''CREATE TABLE ITEMS
(item_id integer not null, item_name varchar(300),
item_description text, item_category text,
quantity_in_stock integer)'''
cursor.execute(sql_statement)
items = [(101, 'Nik D300', 'Nik D300', 'DSLR Camera', 3),
(102, 'Can 1300', 'Can 1300', 'DSLR Camera', 5),
(103, 'gPhone 13S', 'gPhone 13S', 'Mobile', 10),
(104, 'Mic canvas', 'Mic canvas', 'Tab', 5),
(105, 'SnDisk 10T', 'SnDisk 10T', 'Hard Drive', 1)
]
try:
cursor.executemany("Insert into ITEMS values (?,?,?,?,?)", items)
conn.commit()
#Add code to select items here
sql_statement= "select * from ITEMS where item_id<103 "
cursor.execute(sql_statement)
except:
return 'Unable to perform the transaction.'
rowout=[]
for row in cursor.fetchall():
rowout.append(row)
return rowout
conn.close()
'''For testing the code, no input is required'''
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == "__main__":
f = open(os.environ['OUTPUT_PATH'], 'w')
res = main();
f.write(str(res) + "\n")
f.close()
Question 4: Database Connectivity 4 - Update records.
Solution 4:-
#!/bin/python3
import sys
import os
import sqlite3
# Complete the function below.
def main():
conn = sqlite3.connect('SAMPLE.db')
cursor = conn.cursor()
cursor.execute("drop table if exists ITEMS")
sql_statement = '''CREATE TABLE ITEMS
(item_id integer not null, item_name varchar(300),
item_description text, item_category text,
quantity_in_stock integer)'''
cursor.execute(sql_statement)
items = [(101, 'Nik D300', 'Nik D300', 'DSLR Camera', 3),
(102, 'Can 1300', 'Can 1300', 'DSLR Camera', 5),
(103, 'gPhone 13S', 'gPhone 13S', 'Mobile', 10),
(104, 'Mic canvas', 'Mic canvas', 'Tab', 5),
(105, 'SnDisk 10T', 'SnDisk 10T', 'Hard Drive', 1)
]
try:
cursor.executemany("Insert into ITEMS values (?,?,?,?,?)", items)
#Add code for updating quantity_in_stock
sql_statement="update ITEMS set quantity_in_stock=? where item_id= ?"
new_quantity=[(4,103),(2,101),(0,105)]
cursor.executemany(sql_statement, new_quantity)
cursor.execute("select item_id,quantity_in_stock from ITEMS")
except:
'Unable to perform the transaction.'
rowout=[]
for row in cursor.fetchall():
rowout.append(row)
return rowout
conn.close()
'''For testing the code, no input is required'''
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == "__main__":
f = open(os.environ['OUTPUT_PATH'], 'w')
res = main();
f.write(str(res) + "\n")
f.close()
Question 5: Database Connectivity 5 - Delete records
Solution 5:-
#!/bin/python3
import sys
import os
import sqlite3
# Complete the function below.
def main():
conn = sqlite3.connect('SAMPLE.db')
cursor = conn.cursor()
cursor.execute("drop table if exists ITEMS")
sql_statement = '''CREATE TABLE ITEMS
(item_id integer not null, item_name varchar(300),
item_description text, item_category text,
quantity_in_stock integer)'''
cursor.execute(sql_statement)
items = [(101, 'Nik D300', 'Nik D300', 'DSLR Camera', 3),
(102, 'Can 1300', 'Can 1300', 'DSLR Camera', 5),
(103, 'gPhone 13S', 'gPhone 13S', 'Mobile', 10),
(104, 'Mic canvas', 'Mic canvas', 'Tab', 5),
(105, 'SnDisk 10T', 'SnDisk 10T', 'Hard Drive', 1)
]
try:
cursor.executemany("Insert into ITEMS values (?,?,?,?,?)", items)
cursor.executemany("update ITEMS set quantity_in_stock = ? where item_id = ?",
[(4, 103),
(2, 101),
(0, 105)])
#Add code below to delete items
sql_statement= "delete from ITEMS where item_id=105 "
cursor.execute(sql_statement)
cursor.execute("select item_id from ITEMS")
except:
return 'Unable to perform the transaction.'
rowout=[]
for row in cursor.fetchall():
rowout.append(row)
return rowout
conn.close()
'''For testing the code, no input is required'''
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == "__main__":
f = open(os.environ['OUTPUT_PATH'], 'w')
res = main();
f.write(str(res) + "\n")
f.close()
LAB 8: Give a Try - Database Connectivity : : Welcome to Python - Database Connectivity.
Give a Try - Database Connectivity : Welcome to Python - Database Connectivity.
Solution: Give a Try - Database Connectivity : : Welcome to Python - Database Connectivity.
#!/bin/python3
import sys
import os
# Add Celsius class implementation below.
class Celsius:
def __get__(self , object_of_Temperature , owner_Temperature ):
return 5 * (object_of_Temperature.fahrenheit - 32) / 9
def __set__(self, object_of_Temperature, celsius):
object_of_Temperature.fahrenheit = 32 + 9 * celsius / 5
# Add temperature class implementation below.
class Temperature:
celsius = Celsius()
def __init__(self, user_fahrenheit):
self.fahrenheit = user_fahrenheit
'''Check the Tail section for input/output'''
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == "__main__":
with open(os.environ['OUTPUT_PATH'], 'w') as fout:
res_lst = list()
t1 = Temperature(int(input()))
res_lst.append((t1.fahrenheit, t1.celsius))
t1.celsius = int(input())
res_lst.append((t1.fahrenheit, t1.celsius))
fout.write("{}\n{}".format(*res_lst))
LAB 9 : : Welcome to Python Files -- Give a Try PDH # 1 Welcome to Python Files
Question 1: Give a Try PDH # 1 Welcome to Python Files
Solution 1:
#!/bin/python3
import os
import sys
#
#
def multiStr():
zenPython = '''The Zen of Python, by Tim Peters
Beautiful is better than ugly.
Explicit is better than implicit.
Simple is better than complex.
Complex is better than complicated.
Flat is better than nested.
Sparse is better than dense.
Readability counts.
Special cases aren't special enough to break the rules.
Although practicality beats purity.
Errors should never pass silently.
Unless explicitly silenced.
In the face of ambiguity, refuse the temptation to guess.
There should be one-- and preferably only one --obvious way to do it.
Although that way may not be obvious at first unless you're Dutch.
Now is better than never.
Although never is often better than *right* now.
If the implementation is hard to explain, it's a bad idea.
If the implementation is easy to explain, it may be a good idea.
Namespaces are one honking great idea -- let's do more of those!
'''
return (zenPython)
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == "__main__":
f = open(os.environ['OUTPUT_PATH'], 'w')
res = multiStr();
f.write(res + "\n")
f.close()
Question 2
Solution 2:
#!/bin/python3
import os
import sys
import io
#
def main():
zenPython = '''The Zen of Python, by Tim Peters
Beautiful is better than ugly.
Explicit is better than implicit.
Simple is better than complex.
Complex is better than complicated.
Flat is better than nested.
Sparse is better than dense.
Readability counts.
Special cases aren't special enough to break the rules.
Although practicality beats purity.
Errors should never pass silently.
Unless explicitly silenced.
In the face of ambiguity, refuse the temptation to guess.
There should be one-- and preferably only one --obvious way to do it.
Although that way may not be obvious at first unless you're Dutch.
Now is better than never.
Although never is often better than *right* now.
If the implementation is hard to explain, it's a bad idea.
If the implementation is easy to explain, it may be a good idea.
Namespaces are one honking great idea -- let's do more of those!
'''
#Create fp implementation, check for fp instance and return status
fp = io.StringIO(zenPython)
return fp
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == "__main__":
f = open(os.environ['OUTPUT_PATH'], 'w')
res = main();
f.write(str('io.StringIO' in str(res)) + "\n")
f.close()
Question 3
Solution 3:-
#!/bin/python3
import os
import sys
import io
#
# Complete the function below.
def main():
zenPython = '''
The Zen of Python, by Tim Peters
Beautiful is better than ugly.
Explicit is better than implicit.
Simple is better than complex.
Complex is better than complicated.
Flat is better than nested.
Sparse is better than dense.
Readability counts.
Special cases aren't special enough to break the rules.
Although practicality beats purity.
Errors should never pass silently.
Unless explicitly silenced.
In the face of ambiguity, refuse the temptation to guess.
There should be one-- and preferably only one --obvious way to do it.
Although that way may not be obvious at first unless you're Dutch.
Now is better than never.
Although never is often better than *right* now.
If the implementation is hard to explain, it's a bad idea.
If the implementation is easy to explain, it may be a good idea.
Namespaces are one honking great idea -- let's do more of those!
'''
fp = io.StringIO(zenPython)
#Add Implementation step here
zenlines = fp.readlines()
return zenlines[0:5]
'''For testing the code, no input is to be provided'''
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == "__main__":
f = open(os.environ['OUTPUT_PATH'], 'w')
res = main();
f.write(str(res) + "\n")
f.close()
Question 4:
Solution 4:-
#!/bin/python3
import os
import sys
import io
#
# Complete the function below.
def main():
zenPython = '''
The Zen of Python, by Tim Peters
Beautiful is better than ugly.
Explicit is better than implicit.
Simple is better than complex.
Complex is better than complicated.
Flat is better than nested.
Sparse is better than dense.
Readability counts.
Special cases aren't special enough to break the rules.
Although practicality beats purity.
Errors should never pass silently.
Unless explicitly silenced.
In the face of ambiguity, refuse the temptation to guess.
There should be one-- and preferably only one --obvious way to do it.
Although that way may not be obvious at first unless you're Dutch.
Now is better than never.
Although never is often better than *right* now.
If the implementation is hard to explain, it's a bad idea.
If the implementation is easy to explain, it may be a good idea.
Namespaces are one honking great idea -- let's do more of those!
'''
fp = io.StringIO(zenPython)
zenlines = fp.readlines()
#Add implementation step here
zenlines = [ line.strip() for line in zenlines ]
return zenlines
'''For testing the code, no input is to be provided'''
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == "__main__":
f = open(os.environ['OUTPUT_PATH'], 'w')
res = main();
f.write(str(res) + "\n")
f.close()
Question 5:
Solution 5:-
#!/bin/python3
import os
import sys
import io
import re
#
# Complete the function below.
def main():
zenPython = '''
The Zen of Python, by Tim Peters
Beautiful is better than ugly.
Explicit is better than implicit.
Simple is better than complex.
Complex is better than complicated.
Flat is better than nested.
Sparse is better than dense.
Readability counts.
Special cases aren't special enough to break the rules.
Although practicality beats purity.
Errors should never pass silently.
Unless explicitly silenced.
In the face of ambiguity, refuse the temptation to guess.
There should be one-- and preferably only one --obvious way to do it.
Although that way may not be obvious at first unless you're Dutch.
Now is better than never.
Although never is often better than *right* now.
If the implementation is hard to explain, it's a bad idea.
If the implementation is easy to explain, it may be a good idea.
Namespaces are one honking great idea -- let's do more of those!
'''
fp = io.StringIO(zenPython)
zenlines = fp.readlines()
zenlines = [ line.strip() for line in zenlines ]
#Add implementation here to set and match patterns
#Add portions implementation here
portions=re.findall(r"[-*] ?([^-*].*?) ?[-*]",zenPython)
return portions
'''For testing the code, no input is to be provided'''
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == "__main__":
f = open(os.environ['OUTPUT_PATH'], 'w')
res = main();
f.write(str(res) + "\n")
f.close()
Lab 10: Welcome to Python Files - Give a Try PDH # 2 Match pattern and replace
Lab 2 : : Welcome to Python Files -- Give a Try PDH # 2 Match pattern and replace
#!/bin/python3
import os
import sys
import io
import re
#
# Complete the function below.
def subst(pattern, replace_str, string):
#susbstitute pattern and return it
return re.sub(pattern,replace_str,string)
def main():
addr = ['100 NORTH MAIN ROAD',
'100 BROAD ROAD APT.',
'SAROJINI DEVI ROAD',
'BROAD AVENUE ROAD']
#Create pattern Implementation here
#Use subst function to replace 'ROAD' to 'RD.',Store as new_address
p= r"\bROAD\b"
r="RD."
s=",".join(addr)
print(s)
new_address=subst(p,r,s)
return new_address.split(",")
'''For testing the code, no input is required'''
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == "__main__":
f = open(os.environ['OUTPUT_PATH'], 'w')
res = main();
f.write(str(res) + "\n")
f.close()