Kindly Note: If you had any problems, please comment below.
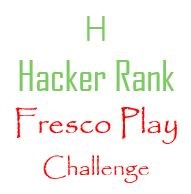
Fresco Play Functions and OOPs Hacker Rank Hands On
LAB 1: Python3 Functions and OOPs | 1 | String Methods
LAB 1 : Python 3 : : Functions and OOPs | 1 | String Methods
# LAB 1 : Python 3 - Functions and OOPs | 1 | String Methods
#!/bin/python3
import math
import os
import random
import re
import sys
def stringmethod(para, special1, special2, list1, strfind):
# Task 1 : Remove all the special characters from "para" specified in "special1" and save them in the variable "word1"
word1=re.sub(f"[{special1}]", "", para)
# Task 2 : Get the first 70 characters from "word1", reverse the string, save it in variable "rword2", and print it.
#rword2=word1[69::-1]
rword2=word1[:70][::-1]
print(rword2)
# Task 3 : Remove all the wide spaces from "rword2", join the character using the special character specified in "special2", and print the joint string.
temp=rword2.replace(" ","").strip()
temp=special2.join(temp)
print(temp)
#Task 4 : If every string from list1 is present in para, then formate the print statement as follow:
# Every string in {list1} were present
# else
# Every String in {list1} were not present
if all ( list1_item in para for list1_item in list1) :
print(f"Every string in {list1} were present")
else:
print(f"Every string in {list1} were not present")
# Task 5 : Split every word from word1 and print the first 20 strings as a list.
s=word1.split()
print(s[:20])
#Task 6 : Calculate the less frequently used words whose count <3 , and print the last 20 less frequent words as a list.
# Note: Count the words in the order as a list.
unique_list=[]
final_list=[]
for i in s:
if i not in unique_list:
unique_list.append(i)
for i in unique_list:
if s.count(i)<3:
final_list.append(i)
print(final_list[-20:])
#Task 7 : Print the last index in word1, where the substring strfind is found.
print(word1.rindex(strfind))
# Refer '__main__' method code which is given below if required.
if __name__ == '__main__':
# Starting from here:
if __name__ == '__main__':
para = input()
spch1 = input()
spch2 = input()
qw1_count = int(input().strip())
qw1 = []
for _ in range(qw1_count):
qw1_item = input()
qw1.append(qw1_item)
strf = input()
stringmethod(para, spch1, spch2, qw1, strf)
LAB 2 Welcome to Python3 - Functions and OOPs | 2 | Magic Constant Generator
LAB 2 : : Welcome to Python 3 -Functions and OOPs | 2 | Magic Constant Generator
#!/bin/python3
import math
import os
import random
import re
import sys
#
# Complete the 'Magic_const' function below.
#
#
#
# The function accepts INTEGER n1 as parameter.
#
def generator_Magic(n1):
# Write your code here
for i in range(3,n1+1):
yield i*((i**2)+1)/2
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == '__main__':
n = int(input().strip())
for i in generator_Magic(n):
print(int(i))
gen1 = generator_Magic(n)
print(type(gen1))
LAB 3 Welcome to Python3 Functions and OOPs | 3 | Prime Number Generator
LAB 3 : : Welcome to Python 3 Functions and OOPs | 3 | Prime Number Generator
# LAB 3 - Welcome to Python 3 Functions and OOPs - Prime Number Generator
#!/bin/python3
import math
import os
import random
import re
import sys
# Complete the 'primegenerator' function below.
def primegenerator(num, val):
# Write your code here
#Task 1: Generate - The prime number for the "num" value of 21 are:-
# o 2,3,5,7,11,13,17,19
k=[]
n2=num
for i in range(2,n2) :
if i <6 and i>1 :
if i !=4 :
k.append(i)
continue
n=int(i/2) # Dividing the number "i" with 2, it will increase the code performace and time execution speed.
condition= False
for j in range(2,n,) :
if i%j==0:
condition=False
break
else:
condition= True
if condition: # Execute If Condition is True
k.append(i)
#Task 2:
# If the value of "val" is 0, then you should yield the following values only:
# o 3,7,13,19
if val==1:
for y in k[::2]:
yield y
# Task 3: If the value of "val" is 1, then you should yield the following values only:
# o 2,5,11,17
if val==0:
for y in k[1::2]:
yield y
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == '__main__':
num = int(input().strip()) # Enter any number, Ex: 30
val = int(input().strip()) # Enter either 0 or 1 only, Ex: 1
for i in primegenerator(num, val):
print(i,end=" ")
LAB 4 Python 3 - Functions and OOPs | 4 | Classes and Objects 1 - [Task 1]
LAB 4 : : Python3 - Functions and OOPs 4 Classes and Objects 1 - [Task 1]
#!/bin/python3
import math
import os
import random
import re
import sys
#
# Complete the 'Magic_const' function below.
#
# Task 1: Define a class "Movie" and Define the initializer method, __init__, that takes three values, Name of the Movie(name), Number of Tickets(n), and the Total Cost (cost), of a movie ticket printing machines, respectively.
class Movie:
def __init__(self,name ,n,cost):
self.name=name
self.n=n
self.cost=cost
# Task 2: Define the method, __str__, inside the class "Movie" and improvise the class definition of "Movie" such that Movie's object displayed in the following formate.
# Movie : Kabir Singh
# Numbers of Tickets : 5
# Total Cost : 666
def __str__(self):
return f"Movie : {self.name}\nNumber of Tickets : {self.n}\nTotal Cost : {self.cost}"
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == '__main__':
name = input()
n = int(input().strip())
cost = int(input().strip())
p1 = Movie(name,n,cost)
print(p1)
LAB 4 Python 3 - Functions and OOPs |4| Classes and Objects 1 - [Task 2]
LAB 4 : : Python 3 - Functions and OOPs | 4 | Classes and Objects 1 - [Task 2]
#!/bin/python3
import math
import os
import random
import re
import sys
# Complete the 'Magic_const' function below.
#
# Task 1: Define the class "comp" and define the initializer method, __init__, that takes 2 values and assign them "Real" and "Imaginary" attributes, respectively.
class comp:
def __init__(self, Real, Imaginary):
self.Real=Real
self.Imaginary=Imaginary
#Task 2: Define a method "add" inside the class "comp", which determines the sum of two complex numbers and Print the sum as "Sum of the two Complex numbers :35+47i".
def add(self,object_p2):
p1_Real = self.Real
p1_Imaginary = self.Imaginary
p2_Real = object_p2.Real
p2_Imaginary = object_p2.Imaginary
print(f"Sum of the two Complex numbers :{p1_Real+p2_Real}+{p1_Imaginary+p2_Imaginary}i")
#Task 3 : Define a method "sub" inside the class "comp", which determines the difference of two complex numbers and Print the difference as "Subtraction of the two Complex numbers :33+43i".
def sub(self,object_p2):
p1_Real = self.Real
p1_Imaginary = self.Imaginary
p2_Real = object_p2.Real
p2_Imaginary = object_p2.Imaginary
sign=""
diff = p1_Imaginary - p2_Imaginary
if diff<0:
sign=f"-{abs(diff)}"
else :
sign=f"+{diff}"
print(f"Subtraction of the two Complex numbers :{p1_Real-p2_Real}{sign}i")
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == '__main__':
Real1 = int(input().strip())
Imaginary1 = int(input().strip())
Real2 = int(input().strip())
Imaginary2 = int(input().strip())
p1 = comp(Real1,Imaginary1)
p2 = comp(Real2,Imaginary2)
p1.add(p2)
p1.sub(p2)
LAB 5 Python 3 - Functions and OOPs 5 Classes and Objects 2 - [Task 1]
LAB 5 : : Python 3 - Functions and OOPs | 5 | Classes and Objects 2 - [Task 1]
#!/bin/python3
import math
import os
import random
import re
import sys
#Start from here
#
class parent:
def __init__(self,total_asset):
self.total_asset = total_asset
def display(self):
print("Total Asset Worth is "+str(self.total_asset)+" Million.")
print("Share of Parents is "+str(round(self.total_asset/2,2))+" Million.")
# It is expected to create two child classes 'son' and 'daughter' for the above class 'parent'
#
#Write your code here
class son(parent):
def __init__(self,t,sp):
parent.__init__(self,t)
self.son_Percentage=round(((t *sp)/100),2)
def son_display(self):
print("Share of Son is {} Million.".format(self.son_Percentage))
class daughter(parent):
def __init__(self,t,dp):
parent.__init__(self,t)
self.daughter_Percentage=round(((t *dp)/100),2)
def daughter_display(self):
print(f"Share of Daughter is {self.daughter_Percentage} Million.")
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == '__main__':
t = int(input())
sp = int(input())
dp = int(input())
obj1 = parent(t)
obj2 = son(t,sp)
obj2.son_display()
obj2.display()
obj3 = daughter(t,dp)
obj3.daughter_display()
obj3.display()
print(isinstance(obj2,parent))
print(isinstance(obj3,parent))
print(isinstance(obj3,son))
print(isinstance(obj2,daughter))
LAB 5 Python 3 Functions and OOPs 5 Classes and Objects 2 - [Task 2]
LAB 5 : : Python 3 Functions and OOPs | 5 | Classes and Objects 2 - [Task 2]
#!/bin/python3
import math
import os
import random
import re
import sys
#
# Task 1: Define a class "rectangle" with two methods "display" and "area" and perform the following operations.
# (a) Define the method "display" and print the message "This is a Rectangle" upon calling.
# (b) Define the method "area" which takes two parameters "Length" and "Breadth" and find the area of the rectangle and print as "Area of Rectangle is 144"
class rectangle:
def area(self,Length,Breadth):
area= Length*Breadth
print(f"Area of Rectangle is {area}")
def display(self):
print("This is a Rectangle")
# Task 2: Define a class "square" with two methods "display" and "area" and perform the following operations.
# (a) Define the method "display" and print the message "This is a Square" upon calling.
# (b) Define the method "area" which takes a single parameters "Length" and "Breadth" and find the area of the square and print as "Area of square is 233"
class square:
def area(self,Side):
area= Side**2
print(f"Area of square is {area}")
def display(self):
print("This is a Square")
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == '__main__':
l = int(input())
b = int(input())
s = int(input())
obj1 = rectangle()
obj1.display()
obj1.area(l,b)
obj2 = square()
obj2.display()
obj2.area(s)
LAB 6 Python 3 - Functions and OOPs 6 Handling Exceptions 1
LAB 6: : Python 3 - Functions and OOPs | 6 | Handling Exceptions 1
#!/bin/python3
import math
import os
import random
import re
import sys
#Task 1: Write the function "Handle_Exc1", that takes no parameters and will handle the "ValueError" exception.
def Handle_Exc1():
#Task 2: Read two integers say a and b from STDIN.
a= int(input())
b= int(input())
#Task 3: Perform the following operations:-
# (a) IF: Raise "ValueError" exception if a is greater than "150" or if b is less than "100" and print a message "Their sum is out of range."
# (b) Else IF : Raise "ValueError" exception if their sum is greater than "400" and print "Their sum is out of range".
# (c) If none of above Exceptions happens, then print "All in range".
if a >150 or b<100:
raise ValueError("Input integers value out of range.")
elif (a+b)>400:
raise ValueError("Their sum is out of range")
else:
print("All in range")
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == '__main__':
try:
Handle_Exc1()
except ValueError as exp:
print(exp)
LAB 7 Welcome to Python 3 Functions and OOPs | 7 | Handling Exceptions 2
LAB 7 : : Welcome to Python 3 Functions and OOPs | 7 | Handling Exceptions 2
#!/bin/python3
import math
import os
import random
import re
import sys
#
# Task1 : Write the function definition for the function "FORLoop", which takes no parameters, as follows:
# (a) Get an Integer input "n" from the user.
# (b) Create a LIST of "n" integers from
#
def FORLoop():
# Write your code here
n= int(input())
l1= [ int(input()) for i in range(n) ]
print(l1)
iter1=iter(l1)
for i in range(n):
print(next(iter1))
return iter1
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == '__main__':
try:
d = FORLoop()
print(type(d))
print(next(d))
except StopIteration:
print('Stop Iteration : No Next Element to fetch')
LAB 8 Welcome to Python 3 Functions and OOPs | 8 | Handling Exceptions 3
LAB 8 : : Welcome to Python 3 Functions and OOPs | 8 | Handling Exceptions 3
#!/bin/python3
import math
import os
import random
import re
import sys
#
# Define the Class for user-defined exceptions "MinimumDepositError" and "MinimumBalanceError" here
#
class MinimumDepositError(Exception):
def __init__(self, value):
self.value = value
def __str__(self):
return str(self.value)
class MinimumBalanceError(Exception):
def __init__(self, value):
self.value = value
def __str__(self):
return str(self.value)
def Bank_ATM(balance,choice,amount):
# Write your code here
bal=balance
ch=choice
if balance<500:
raise ValueError("As per the Minimum Balance Policy, Balance must be at least 500")
if ch==1:
if amount<2000:
raise MinimumDepositError("The Minimum amount of Deposit should be 2000.")
else:
bal+=amount
print(f"Updated Balance Amount: {bal}")
elif ch==2:
bal= bal-amount
if bal<500:
raise MinimumBalanceError("You cannot withdraw this amount due to Minimum Balance Policy")
else:
print(f"Updated Balance Amount: {bal}")
else:
pass
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == '__main__':
bal = int(input())
ch = int(input())
amt = int(input())
try:
Bank_ATM(bal,ch,amt)
except ValueError as e:
print(e)
except MinimumDepositError as e:
print(e)
except MinimumBalanceError as e:
print(e)
LAB 9 Welcome to Python 3 Functions and OOPs | 9 | Handling Exceptions 4
LAB 9 : : Welcome to Python 3 Functions and OOPs | 9 | Handling Exceptions 4
#!/bin/python3
import math
import os
import random
import re
import sys
#
# Tassk 1: This is a typical Interface privided in the library, which takes 3 inputs from the library members, sequentially.
# They are:
# o memberfee,
# o installment,
# o book
#
def Library(memberfee,installment,book):
avail_book=["philosophers stone", "chamber of secrets","prisoner of azkaban", "goblet of fire", "order of phoenix","half blood prince","deathly hallows 1","deathly hallows 2"]
# Task 2: Raise "ValueError" exception if the input for the number of installments is greater than "3". And printa message "Maximum Permitted Number of Installements is 3"
if installment > 3:
raise ValueError("Maximum Permitted Number of Installments is 3")
# Task 3: Raise 'ZeroDivisionError' exception if the input for the number of installments is equal to "0" and print a message "Number of installments cannot be Zero."
# else : Print the amount per installment as "Amount per installment is 3000.0".
if installment ==0:
raise ZeroDivisionError("Number of Installments cannot be Zero.")
else:
amt_per_install= memberfee/installment
print(f"Amount per Installment is {amt_per_install}")
# Task 4: Raise "NameErro" exception if the user is looking for a book other than the books mentioned above and print a messsage "No such books exists in this section".
# else: print "It is available in this section".
if book not in avail_book:
raise NameError("No such book exists in this section")
else:
print("It is available in this section")
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == '__main__':
memberfee = int(input())
installment = int(input())
book = input()
try:
Library(memberfee,installment,book)
except ZeroDivisionError as e:
print(e)
except ValueError as e:
print(e)
except NameError as e:
print(e)
LAB 10 Functions and OOPs |10| Modules 1 - DateTime
LAB 10 : : Functions and OOPs |10| Modules 1 - DateTime
#!/bin/python3
import math
import os
import random
import re
import sys
import datetime
#
# Complete the 'dateandtime' function below.
#
# The function accepts INTEGER val as parameter.
# The return type must be LIST.
#
# Note: DateTime Module needed to import for completion this LAB.
def dateandtime(val,tup):
# Write your code here
l=[]
if val==1:
d=datetime.date(tup[0],tup[1],tup[2])
p=d.strftime("%d/%m/%Y")
l.append(d)
l.append(p)
elif val== 2:
tup=int(''.join(map(str, tup)))
date_time=datetime.date.fromtimestamp(tup)
l.append(date_time)
elif val==3:
t=datetime.time(tup[0],tup[1],tup[2])
l.append(t)
l.append(t.strftime("%I"))
elif val==4:
Day_Month_YearInDays=["%A", "%B", "%j"]
inp= tup #(2017, 11, 18)
dt = datetime.datetime.strptime(f"{inp[0]}/{inp[1]}/{inp[2]}", "%Y/%m/%d")
l=list(map(lambda x: dt.strftime(x),Day_Month_YearInDays))
elif val==5:
d=datetime.date(tup[0],tup[1],tup[2])
t=datetime.time(tup[3],tup[4],tup[5])
z=datetime.datetime.combine(d,t)
l.append(z )
return l
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == '__main__':
val = int(input().strip())
if val ==1 or val==4 or val ==3:
qw1_count=3
if val==2:
qw1_count=1
if val ==5:
qw1_count=6
qw1 = []
for _ in range(qw1_count):
qw1_item = int(input().strip())
qw1.append(qw1_item)
tup=tuple(qw1)
ans = dateandtime(val,tup)
print(ans)
LAB 11 Python 3 - Functions and OOPs |11| Modules 2 - Itertools
LAB 11 : : Python 3 - Functions and OOPs | 11 | Modules 2 - Itertools
#!/bin/python3
import math
import os
import random
import re
import sys
import import itertools
#
# Complete the 'usingiter' function below.
#
# The function is expected to return a TUPLE.
# The function accepts following parameters:
# 1. TUPLE tupb
#
def performIterator(tuplevalues):
# Write your code here
t= tuplevalues
temp=[]
b=[]
y=itertools.cycle(t[0])
for i in range(length):
if i >3:
break
temp.append(next(y))
b.append(tuple(temp))
#Task 2:
t= tuplevalues
temp.clear()
t=itertools.repeat(t[1][0],length)
for i in range(length):
temp.append(next(t))
b.append(tuple(temp))
#b.append(tuple([ next(t) for i in range(length)]))
#Task 3:
import operator
t= tuplevalues
temp.clear()
j=itertools.accumulate(t[2], operator.add)
for i in j:
temp.append(i)
b.append(tuple(temp))
#b.append(tuple([i for i in j]))
#Task 4
t= tuplevalues
temp.clear()
t=itertools.chain(*t)
for i in t:
temp.append(i)
b.append(tuple(temp))
#Task 5
temp2=temp.copy()
t=itertools.filterfalse(lambda x : x%2==0,temp2)
temp.clear()
for i in t:
temp.append(i)
# print(i)
b.append(tuple(temp))
b=tuple(b)
return b
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == '__main__':
length = int(input().strip())
qw1 = []
for i in range(4):
qw2 = []
for _ in range(length):
qw2_item = int(input().strip())
qw2.append(qw2_item)
qw1.append(tuple(qw2))
tupb = tuple(qw1)
q = performIterator(tupb)
print(q)
LAB 12 Python 3 - Functions and OOPs 12 Modules 3 -- Cryptography
LAB 12 : : Python 3 - Functions and OOPs | 12 | Modules 3 -- Cryptography
#!/bin/python3
import math
import os
import random
import re
import sys
from cryptography.fernet import Fernet
#
# Complete the 'encrdecr' function below.
#
# The function is expected to return a LIST.
# The function accepts following parameters:
# 1. STRING keyval
# 2. STRING textencr
# 3. Byte-code textdecr
#
def encrdecr(keyval, textencr, textdecr):
# Write your code here
l=[]
fernet =Fernet(keyval)
encMessage = fernet.encrypt(textencr)
l.append(encMessage)
decMessage = fernet.decrypt(textdecr)
l.append(decMessage.decode())
return l
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == '__main__':
fptr = open(os.environ['OUTPUT_PATH'], 'w')
file = open('key.key', 'rb')
key = file.read() # The key will be type bytes
file.close()
keyval = key
textencr = str(input()).encode()
textdecr = str(input()).encode()
result = encrdecr(keyval, textencr, textdecr)
bk=[]
f = Fernet(key)
val = f.decrypt(result[0])
bk.append(val.decode())
bk.append(result[1])
fptr.write(str(bk) + '\n')
fptr.close()
LAB 13 Python 3 - Functions and OOPs | 13 | Modules 4 -- Calendar
LAB 13 : : Python 3 - Functions and OOPs | 13 | Modules 4 -- Calendar
#!/bin/python3
import math
import os
import random
import re
import sys
import calendar
from collections import Counter
# Complete the function "usingcalendar" which accepts TUPLE "datetuple" as parameter.
#
def usingcalendar(datetuple):
# Write your code here
#Task 1: Checkwhether the given year from the tuple is a leap year.
# if it is a leap year then assign the month's value as 2 throughout the whole function. And print the monthly calendar for the specified year and month.
year = datetuple[0]
month= datetuple[1]
date = datetuple[2]
if year%4==0: # Checking wheather Leap Year True or False
month=2
obj_Cal= calendar.TextCalendar(calendar.MONDAY) # Fixing the Calendar starts with Monday
calendar_display = obj_Cal.formatmonth(year, month)
print(calendar_display)
#Task 2: Use "itermonthdates" module from the calendar to iterate through the dates of the specified month and year in the calendar. And print the last 7 dates that appears on the calendar of that months as a list.
w=list( obj_Cal.itermonthdates(year, month))
print(w[-7:])
# Task 3: Print the day of the week which appears the most frequent in the specified month and the year.
# If there is more than one frequent day, then consider the one that comes first. Ex: If there are same number of Monday and Tuesday, then consider Monday.
counter_object = Counter(d.strftime('%A') for d in obj_Cal.itermonthdates(year, month) if d.month==month)
common_Days=counter_object.most_common()
print(common_Days[0][0])
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == '__main__':
qw1 = []
for _ in range(3):
qw1_item = int(input().strip())
qw1.append(qw1_item)
tup=tuple(qw1)
usingcalendar(tup)
LAB 14 Python 3 - Functions and OOPs 14 Modules 5 -- Collections
LAB 14 Python 3 - Functions and OOPs | 14 | Modules 5 -- Collections
#!/bin/python3
import math
import os
import random
import re
import sys
import collections
#
# Complete the 'collectionfunc' function below.
#
# The function accepts following parameters:
# 1. STRING text1
# 2. DICTIONARY dictionary1
# 3. LIST key1
# 4. LIST val1
# 5. DICTIONARY deduct
# 6. LIST list1
#
def collectionfunc(text1, dictionary1, key1, val1, deduct, list1):
# Write your code here
d={}
# Task 1
for i in text1.split():
if i in d:
d[i]+=1
else:
d[i]= 1
sort_d= dict(sorted(d.items(),key= lambda kv:kv[0]))
print(sort_d)
# Task 2
cou= collections.Counter(dictionary1)
for each in deduct:
cou[each]-=deduct[each]
# print(dict(cou))
print(dict(cou))
# print("yes 2")
# Task 3
orde=collections.OrderedDict()
for i in range(len(key1)):
orde[key1[i]]= val1[i]
del orde [key1[1]]
# print("yes")
orde[key1[1]]=val1[1]
print(dict(orde))
# Task 4
d4={ "odd":[],"even":[]}
for i in list1:
if i%2==0:
temp=d4["even"]
temp.append(i)
d4["even"]=temp
else:
temp =d4["odd"]
temp.append(i)
d4["odd"]=temp
if d4["even"] ==[]:
del d4["even"]
if d4["odd"]==[]:
del d4["odd"]
print(d4)
# Refer '__main__' method code which is given below if required.
# if __name__ == '__main__':
# Starting from here:
if __name__ == '__main__':
text1 = input()
n1 = int(input().strip())
qw1 = []
qw2 = []
for _ in range(n1):
qw1_item = (input().strip())
qw1.append(qw1_item)
qw2_item = int(input().strip())
qw2.append(qw2_item)
testdict={}
for i in range(n1):
testdict[qw1[i]]=qw2[i]
collection1 = (testdict)
qw1 = []
n2 = int(input().strip())
for _ in range(n2):
qw1_item = (input().strip())
qw1.append(qw1_item)
key1 = qw1
qw1 = []
n3 = int(input().strip())
for _ in range(n3):
qw1_item = int(input().strip())
qw1.append(qw1_item)
val1 = qw1
n4 = int(input().strip())
qw1 = []
qw2 = []
for _ in range(n4):
qw1_item = (input().strip())
qw1.append(qw1_item)
qw2_item = int(input().strip())
qw2.append(qw2_item)
testdict={}
for i in range(n4):
testdict[qw1[i]]=qw2[i]
deduct = testdict
qw1 = []
n5 = int(input().strip())
for _ in range(n5):
qw1_item = int(input().strip())
qw1.append(qw1_item)
list1 = qw1
collectionfunc(text1, collection1, key1, val1, deduct, list1)