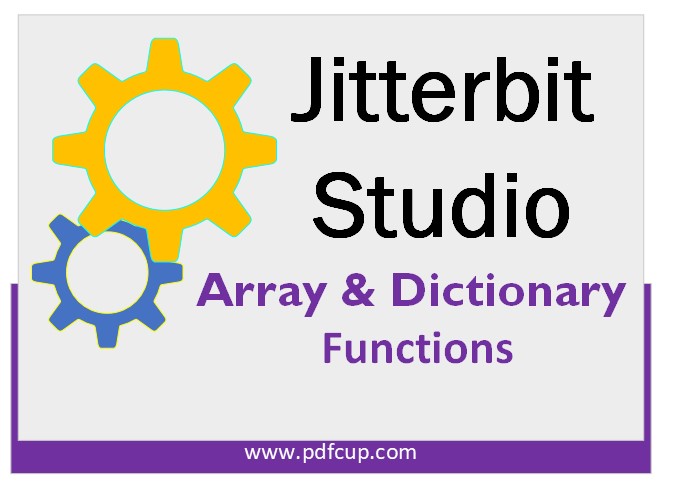
Also Read, How to Download Jitterbit Studio in Windows, Linux, and Mac.
1. Dict():
The Jitterbit Dict() function is used to build an empty dictionary. Similar to other programming's dictionary, Jitterbit dictionary exhibits similar behavior. The data is organized into key-value pairs. A dictionary's value may be in any permissible data type, but its key must always be in string or have a string representation.
There are built-in functions for adding, deleting, and changing dictionary data to making the Jitterbit dictionary mutable or dynamic. In contrast to temporary storage, file storage, and global variables, the Jitterbit dictionary can be utilized to store data in memory relatively effectively and with minimal letency.
My_Dictionary_1=Dict();
//Return: {}, An empty Dictionary.
My_Dictionary_2= {}
//Return: {}, An empty Dictionary.
My_Dictionary_1 = Dict();
My_Dictionary_1['a'] = 10;
My_Dictionary_1['b'] = 20;
WriteToOperationLog(My_Dictionary_1);
//Return: [a=>"10",b=>"20"]
//Another way to create a dictionary is to use "{}", but we cannot directly add data to the dictionary as we can with the Dict() function.
temp_Dict = {};
temp_Dict["Rat"] = "Micky Mouse";
WriteToOperationLog(temp_Dict);
//Return: {}
//We must need to use AddToDict() function to add the data into the dictionary if the initilzation start with '{}'.
My_Dictionary_2 = {};
AddToDict(My_Dictionary_2, "Country", "USA");
WriteToOperationLog(My_Dictionary_2);
//Return: [Country=>"USA"]
2. Map():
The Jitterbit Map() function provides another way to create a dictionary, but unlike the Dict() function, we cannot directly add data to the dictionary; instead, we must use AddToDict() to do so.
temp_dictionary = Map();
//Dictionary Declaration
my_dict= Map();
AddToDict(my_dict,"Cat","Tom");
my_dict['Rat']= "Jerry";
WriteToOperationLog(my_dict);
//Return: [Cat=>"Tom",Rat=>"Jerry"]
//The "HasKey" will be extremely useful if we need to update the existing dictionary values.
my_dict_2= Map();
my_dict_2["Rat"]= "Jerry"
//If the first argument is not a dictionary or the key was not found, it returns false, else Update the value.
If(HasKey(my_dict_2, "Rat"), my_dict_2["Rat"]="Micky Mouse");
//Return: [Rat=>"Micky Mouse"]
3. Array():
A list of elements with the same date type are created in an ordered list using the Jitterbit Array() function. The values in an array can be retrieved individually by iterating the array using a loop call as an array does not store values with a "key:value" pair.
// Option 1 to create Array list.
$Marks = Array();
// Option 2 to create Array list.
$Mobile_Stock = {1, 2, 3};
// Create an empty array and set values
arr = Array();
arr[0] = "value1 in index 0";
arr[1] = "value2 in index 1";
//Return: {value1 in index 0,value2 in index 1}
arr = {1, 2, 3};
value = 1;
FindValue(value, arr, arr) == value;
//Return: 1, (true)
value = 4;
FindValue(value, arr, arr) == value;
//Return: 0. (false)
4. HasKey():
The Jitterbit Hashmap() function iterates through each key in the given dictionary until it finds the "key," returning 'True' if the "key" exists and false otherwise. It also helps to prevent the dictionary from overwriting the Key or creating duplicate data. This function allows for updates, deletions, and creations the records.
HasKey(<dict>, <key>);
//The "HasKey" will be extremely useful if we need to update the existing dictionary values.
my_dict_1= Map();
//If the first argument is not a dictionary or the key was not found, it returns false.
If(HasKey(my_dict_1, "Rat"), my_dict_1["Rat"]="Micky Mouse");
//Return: None or Empty or Blank response
// It will check the condition if the Key is not available in Dictionary then it will Add the new KEY and Value. otherwise it will not not perform any activity.
my_dict_2 = Dict();
If(!HasKey(my_dict_2, "Dog"), my_dict_2["Dog"]="Bully");
my_dict_2["Cat"] = "Tom" // Simplest way to add.
//Return: [Cat=>"Tom",Dog=>"Bully"]
//If the Key is not available in Dictionary then it will Add the new KEY and Value, otherwise manual error message will print which may be helpfull to debug the failure condition.
If(!HasKey(my_dict_2, "Dog"), my_dict_2["Dog"]="Bully New", "Condition Failed: Key Already Present in Dictionary");
//Return: Condition Failed: Key Already Present in Dictionary.
5. GetKeys():
The Jitterbit GetKeys() function iterates through each items in the given dictionar and returns only the Keys without its value. The keys return in the array format.
GetKeys(<dict>);
//The "GetKeys" will be extremely useful when we need to fetch only the keys from the existing dictionary.
My_Dictionary_3 = Dict();
My_Dictionary_3['a'] = 10;
My_Dictionary_3['b'] = 20;
//Calling function.
GetKeys(My_Dictionary_3);
//Return: {a, b}
// if the Key is not available in Dictionary then it will not return anything.
my_dict_2 = Dict(); //empty Dictionary
GetKeys(my_dict_2);
//Return: Return Null or empty list.
6. CollectValues():
The Jitterbit CollectValues() function iterates through each items in the given dictionary and returns only the values without its Keys. The Values return in the array format.
CollectValues(<dict>, <namesArray>);
//The "CollectValues" will be extremely useful when we need to fetch only the Values from the existing dictionary.
My_Dictionary_4 = Dict();
My_Dictionary_4['a'] = 100;
My_Dictionary_4['b'] = 200;
//Calling function.
CollectValues(My_Dictionary_4, GetKeys(My_Dictionary_4));
//Return: {100, 200}
// if the Value is not available in Dictionary then it will not return anything except placeholder.
My_Dictionary_4 = Dict();
My_Dictionary_4['a'] = 100;
My_Dictionary_4['b'] = '';
//Calling function.
CollectValues(My_Dictionary_4, GetKeys(My_Dictionary_4));
//Return: {100,}
7. SortArray():
The Jitterbit SortArray() function iterates through each items in the given Array and returns the sorted(Ascending or Descending) Array List .
SortArray(<arrayToSort>[, <isAscending>])
SortArray(<arrayToSort>, <index>[, <isAscending>])
// Sorting 1-Dimensional Array
firstArray = {"Artificial ", "Intelligent ", "Machine", "Learning"};
SortArray(firstArray);
WriteToOperationLog(firstArray);
//Return: {Artificial ,Intelligent ,Learning,Machine}
// We can also add Prefix to each elements if needed like this-
firstArray = " 101_"+ firstArray;
WriteToOperationLog(firstArray);
//Return: { 101_Artificial , 101_Intelligent , 101_Learning, 101_Machine}
// We can also give index number as prefix to each array elements like this-
indx=0;
while(indx <Length(firstArray),
firstArray[indx] = " " + indx + "_" + firstArray[indx];
indx = indx+1;
);
WriteToOperationLog(firstArray);
//Return: { 0_Artificial , 1_Intelligent , 2_Learning, 3_Machine}
// Sorting 2-Dimensional array
secondArray = {{"Artificial ", "Intelligent ", "Machine", "Learning", 200},
{"Artificial ", "Intelligent ", "Machine", "Learning", 100},
{"Artificial ", "Intelligent ", "Machine", "Learning", 250},
{"Artificial ", "Intelligent ", "Machine", "Learning", 150}};
// Sort secondArray, order by fifth column's values, and 'false' boolean value is indicating that the Ascending sorting is disabled now.
SortArray(secondArray, 4, false);
/*Return: {{Artificial ,Intelligent ,Machine,Learning,250},
{Artificial ,Intelligent ,Machine,Learning,200},
{Artificial ,Intelligent ,Machine,Learning,150},
{Artificial ,Intelligent ,Machine,Learning,100}}
*/
// We can also fetch single value form 2-D array like this-
secondArray[1][4];
// Return: 200
// We can also implement numeric computation 'Power' on each int-type array elements like this-
i=0;
while(i<Length(secondArray),
secondArray[i][4] = secondArray[i][4]^2;
i=i+1;
);
secondArray;
/*Return: {{Artificial ,Intelligent ,Machine,Learning,62500},
{Artificial ,Intelligent ,Machine,Learning,40000},
{Artificial ,Intelligent ,Machine,Learning,22500},
{Artificial ,Intelligent ,Machine,Learning,10000}}
8. AddToDict():
The Jitterbit AddToDict() function is used to add elements to an existing dictionary. By default, the keys are automatically sorted and arranged in ascending order. There are two ways to define a dictionary in Jitterbit, each offering a different method of implementation, as explained below.
AddToDict(<dict>, <key>, <value>])
My_Dictionary_2 = Dict();
My_Dictionary_2["Rat"] = "Micky Mouse";
AddToDict(My_Dictionary_2, "Country1", "USA");
AddToDict(My_Dictionary_2, "Country2", "India");
AddToDict(My_Dictionary_2, "BallName", "Football");
WriteToOperationLog(My_Dictionary_2);
//Output: [BallName=>"Football",Country1=>"USA",Country2=>"India",Rat=>"Micky Mouse"]
My_Dictionary_2 = {};
My_Dictionary_2["BestCartoon"] = "Micky Mouse"; // This type of assignment will not work if dictionary is declared using curly braces '{}'.
AddToDict(My_Dictionary_2, "Country1", "USA");
AddToDict(My_Dictionary_2, "Country2", "India");
WriteToOperationLog(My_Dictionary_2);
//Output: [Country1=>"USA",Country2=>"India"]
My_Dictionary_3 = Dict();
AddToDict(My_Dictionary_3, "BallName", "Football");
AddToDict(My_Dictionary_3, "EmpID",{100,200,300,400,500});
AddToDict(My_Dictionary_3, "BallName", "VolleyBall"); // Overwrite first value 'Football'
WriteToOperationLog(My_Dictionary_3);
//Output: [BallName=>"VolleyBall",EmpID=>"{100,200,300,400,500}"]
My_Dictionary_3["EmpID"][2] // Access nested elements using the square brackets
//Output: 300
9. RemoveKey():
The Jitterbit RemoveKey() function iterates through each item in the given dictionary and removes or pops only the matched key along with its associated value. This function returns 1 to indicate that the key-value pair was successfully removed and 0 to indicate that the removal of the key-value pair failed.
RemoveKey(<dict>, <Key>);
My_Dictionary_3 = Dict();
//Inserting items
My_Dictionary_3['a'] = 10;
My_Dictionary_3['b'] = 20;
// Direct removing the key without prior checking whether the key exists or not.
result = RemoveKey(My_Dictionary_3, "a");
WriteToOperationLog(result);
WriteToOperationLog(My_Dictionary_3);
My_Dictionary_3 = Dict();
My_Dictionary_3['a'] = 10;
My_Dictionary_3['b'] = 20;
// Best practice to check whether the key exists before removing.
If(HasKey(My_Dictionary_3, "c"),
WriteToOperationLog("Key exists. Removed Successfully!")
result= RemoveKey(My_Dictionary_3, "c"),
WriteToOperationLog("Key doesn't exists.")
);
WriteToOperationLog("result: " + result);
WriteToOperationLog("My_Dictionary_3: " + My_Dictionary_3);