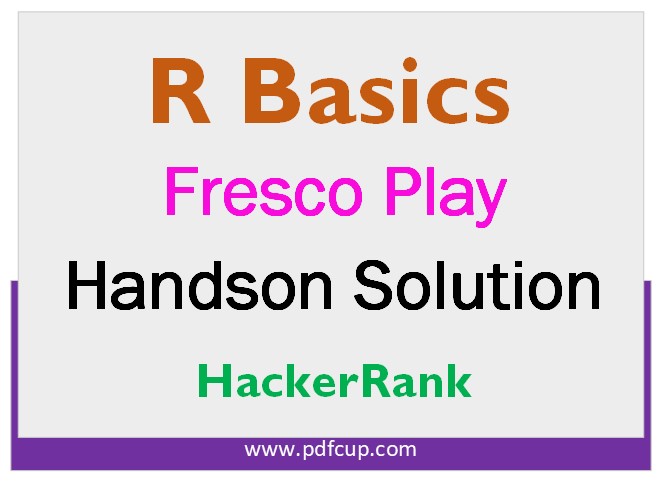
Lab 1: Welcome to R Basics - Factor and Levels
Solution 1: Factor and Levels
# Enter your code here. Read input from STDIN. Print output to STDOUT
vector_list = c("yes","no","yes","maybe")
Factor_list = factor(vector_list)
levels_list = levels(Factor_list)
print(levels_list)
Lab 2: Date and Time in R
Solution 2: Welcome to R Basics - Date and Time in R
Solution:
# Enter your code here. Read input from STDIN. Print output to STDOUT
#str = "2016-12-25"
str = "25-12-2016"
d <- as.Date(str, format="%d-%m-%Y")
print(d)
Lab 3: Vectors in R
Solution 3 : Welcome to R Basics -Vectors in R
Solution:
# Enter your code here. Read input from STDIN. Print output to STDOUT
V1 <- c(1,2,3,4,5)
V2 <- c(10,20,30,40,50)
result <- V1+ V2
print(result)
Lab 4: Vectors and Matrices
Solution : Welcome to R Basics - Vectors and Matrices
Solution:
# Enter your code here. Read input from STDIN. Print output to STDOUT
vector_V <- c(1,2,3,4,5,6,7,8,9)
matrix_M <- matrix(vector_V, byrow = TRUE,nrow=3,ncol=3)*2
print(matrix_M)
Lab 5: Categorical to Numeric Variable in R
Solution 5 : Welcome to R Basics -Categorical to Numeric Variable in R
Solution:
# Enter your code here. Read input from STDIN. Print output to STDOUT
set.seed(10)
v1 <- factor( c(sample.int(100, 10)))
v2 <- as.numeric(as.character(v1))
print(v1==v2)
Lab 6: Random Numbers in R
Solution 6 : Welcome to R Basics - Random Numbers in R
Solution:
random_function <- function(length,start,end,precision) {
# Enter your code here.
V <- round(runif(length, start, end),precision)
return (V)
}#End Function
print(length(random_function(10,0.0,10.0,2)))
Lab 7: Handling 'NA'
Solution 7 : Welcome to R Basics - Handling 'NA'
Solution:
handling_na <- function(V)
{
# Enter your code here. Read input from STDIN. Print output to STDOUT
even_num <- V[ V%%2==0]
# print(even_num)
exclude_NA <- even_num[!is.na(even_num) ]
# print(exclude_NA)
V <- exclude_NA
return (V)
}
vec <-c(1, 4 ,NA ,7 ,9 ,NA ,2)
print(handling_na(vec))
Lab 8: Set Operations in R
Solution 8 : Welcome to R Basics - Set Operations in R
Solution:
set_handling <- function(A,B)
{
# Enter your code here. Read input from STDIN. Print output to STDOUT
C <- setdiff(A,B)
return (C)
}
print(set_handling(c(11:16),c(13:20)))
Lab 9: Descriptive Statistics in R
Solution 9 : Welcome to R Basics - Descriptive Statistics in R
Solution:
stats <- function(V)
{
# Enter your code here. Read input from STDIN. Print output to STDOUT
ans <- median(V)
return (ans)
}
print(stats(c(11:17)))
Lab 10: Conditional Operations - if/else
Solution 10 : Welcome to R Basics - Conditional Operations - if/else
Solution:
conditional <- function(V)
{
# Enter your code here. Read input from STDIN. Print output to STDOUT
for (x in V) {
if (any(x >= 80)) {
cat('Above_Average\t')
k <- c('Above_Average')
} else {
cat('Average\t')
}
}
}
conditional(c(78,85,90))
Lab 11: Conditional Operations - which()
Solution 11 : Welcome to R Basics - Conditional Operations - which()
Solution:
conditional <- function(M)
{
# Enter your code here. Read input from STDIN. Print output to STDOUT
ans <- which(M>13 & M<16)
return(ans)
}
print(conditional( c(10:20)))
Lab 12: for() Loops in R
Solution 12 : Welcome to R Basic -for() Loops in R
Solution:
loop <- function(V) {
# Enter your code here. Read input from STDIN. Print output to STDOUT
new_Vec <- c()
for(i in V){
if( i%%2==0){
# print("even")
# print(i)
new_Vec = append(new_Vec, i)
}
}
# print(sum(f))
ans <- sum(new_Vec)
return (ans)
}
print(loop(c(80:100)))
Lab 13: Writing Functions
Solution 13 : Welcome to R Basics - Writing Functions
Solution:
# Enter your code here. Read input from STDIN. Print output to STDOUT
Cube <- function(num){
return(num**3)
}
print(Cube(10))
print(Cube(3))
print(Cube(4))
print(Cube(5))
Lab 14: Variables
Solution 14 : Welcome to R Basics - Variables
Solution:
leap <-function(D){
# Enter your code here. Read input from STDIN. Print output to STDOUT
ans <-format(D, '%Y')
return (ans)
}
print(leap(as.Date('2018-01-01')))
print(leap(as.Date('2022-01-01')))
Lab 15: Variable Operations
Solution 15 : Welcome to R Basics - Variable Operations
Solution:
operation <-function(L1,L2){
# Enter your code here. Read input from STDIN. Print output to STDOUT
ans <- sqrt(L1)*L2
return (ans)
}
print(operation(4,2))
print(operation(100,4))
Lab 16: Type Conversion
Solution 16 : Welcome to R Basics-Type Conversion
Solution:
convert <-function(K){
# Enter your code here. Read input from STDIN. Print output to STDOUT
type <- typeof(K)
# print(type)
ans <- as.integer(K)
# print(typeof(ans))
return (ans)
}
print(convert(2.7))
print(convert(3.456))
print(convert(4.1))
Lab 17: Factor and Levels
Solution 17 : Welcome to R Basics - Factor and Levels 2
Solution:
level <-function(V){
# Enter your code here. Read input from STDIN. Print output to STDOUT
fac <- factor(V)
ans <- nlevels(fac)
return(ans)
}
print(level(c("Red","Green","Blue")))
print(level(c("Single","Married")))
print(level(c("Apple","Apple","Orange","Mango")))
Lab 18: Random Numbers
Solution 18 : Welcome to R Basics-Random Number 2
Solution:
sequence_gen <-function(a,b){
# Enter your code here. Read input from STDIN. Print output to STDOUT
# ans <- sample(a:b, abs(a-b), replace=FALSE)
ans <- rep(seq(a,b), times = 2)
# print(length(ans))
k <- c()
for(i in ans){
# print(i)
if(i%%2==0){
k<- append(k,i)
}
}
ans <- k
return(ans)
}
print(sequence_gen(10,20))
print(sequence_gen(28,45))
Lab 19: Vector Operations
Solution 19 : Welcome to R Basics - Vector Operations
Solution:
multi <-function(a,b,c,d){
# Enter your code here. Read input from STDIN. Print output to STDOUT
X <- c(a:b)
# print(X)
Y <- c(c:d)
# print(Y)
ans <- X*Y
return(ans)
}
print(multi(1,3,4,6))
print(multi(10,16,24,30))
Lab 20: Matrix Operations
Solution 20 : Welcome to R Basics - Matrix Operations
Solution:
create_mat <-function(V,r,c){
# Enter your code here. Read input from STDIN. Print output to STDOUT
ans <- matrix(V, nrow =r , ncol = c)
return(ans)
}
print(create_mat(c(2,4,6,8,0,9),2,3))
print(create_mat(c(12,14),1,2))
print(create_mat(c(15),1,1))
Lab 21 -::- String and Date
Solution 21 : Welcome to R Basics -String and Date
Solution:
convert_date <-function(S){
# Enter your code here. Read input from STDIN. Print output to STDOUT
dt <- as.Date(S, "%d%m%Y")
ans <- format(dt, "%Y-%m-%d")
return(ans)
}
print(convert_date("15081947"))
print(convert_date("18081995"))
print(convert_date("04021967"))
print(convert_date("06091969"))
Lab 22: POSIX Date
Solution 22 : Welcome to R Basics-POSIX Date
Solution:
elapsed_days <-function(X,Y){
# Enter your code here. Read input from STDIN. Print output to STDOUT
x <- as.POSIXct(X,format="%d%b%Y")
y <- as.POSIXct(Y,format="%d%b%Y")
# print(x)
# print(y)
# using the optional units= argument can be used with any of the following values: "auto", "secs", "mins", "hours", "days", or "weeks".
ans <- difftime(x,y,units='days')
return (ans)
}
print(elapsed_days("15Jan2020","12Dec1983"))
print(elapsed_days("18Aug2021","18Aug1995"))
Lab 23: Conditional Operations
Solution 23 : Welcome to R Basics-Conditional Operations-1
Solution:
condition <-function(temp){
# Enter your code here. Read input from STDIN. Print output to STDOUT
tmp <- temp
for(i in 1:length(temp)){
# print(as.integer(temp[i]))
if(as.integer(temp[i])>100){
temp[i]= "Hot"
}
else{
temp[i]= "Normal"
}
}
# print(temp)
V= temp
return(V)
}
print(condition(c(102,98,67,115)))
print(condition(c(89,125)))
print(condition(c(99,45,56)))
Lab 24: Conditional Operations
Solution 24 :Welcome to R Basics - Conditional Operations-2
Solution:
classmark <-function(marks){
# Enter your code here. Read input from STDIN. Print output to STDOUT
if(any(marks < 90)){
ans <- "Needs Improvement"
}
else{
ans <- "Best Class"
}
return(ans)
}
print(classmark(c(100,95,94,56)))
print(classmark(c(100,95,94,96)))
Lab 25: Iterations
Solution 25 : Welcome to R Basics - Iterations 1
Solution:
# Enter your code here. Read input from STDIN. Print output to STDOUT
Nfact <- function(n){
count <- 1
mul <- 1
while(count < n+1){
# print(i)
mul <- mul*count
count <- count+1
}
return(mul)
}
print(Nfact(6))
print(Nfact(5))
print(Nfact(9))
Lab 26: Iterations
Solution 26 : Welcome to R Basics - Iterations 2
Solution:
# Enter your code here. Read input from STDIN. Print output to STDO
sum_whole <- function(n){
ans <- 0
for(i in 1:n){
ans <- ans+i
}
return(ans)
}
print(sum_whole(20))
print(sum_whole(32))
print(sum_whole(4))
Lab 27: Function - which()
Solution 27 : Welcome to R Basics -Function - which()
Solution:
find_matches <-function(scores){
# Enter your code here. Read input from STDIN. Print output to STDOUT
ans <- which(scores%%2==0 )
return(ans)
}
print(find_matches(c(102,34,56,77)))
print(find_matches(c(100,90,21)))
Lab 28: Function - str()
Solution 28 : Welcome to R Basics-Function - str()
Solution:
inspect <-function(names,details){
# Enter your code here. Read input from STDIN. Print output to STDOUT
# 1. Create a data frame df with the columns from the vectors name, details passed as arguments.
df <- data.frame(names = factor(names), details = factor(details))
# 2. Pass the data frame to the function str() to inspect and print the inspect result.
str(df)
}
inspect(c("Ajay","Ajith","Akhila"),c("Manager","Trainee","Trainee"))
Lab 29: User Defined Functions
Solution 29 : Welcome to R Basics-User Defined Functions
Solution:
# Enter your code here. Read input from STDIN. Print output to STDOUT
pyth <- function(a,b){
c = (a**2) + (b**2)
return(sqrt(c))
}
print(pyth(3,4))
print(pyth(6,8))
Lab 30: R - Programming - Hands-on
Solution 30 : Welcome to R Basics - Test Your Knowledge
Solution:
# Enter your code here. Read input from STDIN. Print output to STDOUT
#Task 1
#Write the function random that accept three parameters (count,l_limit,u_limit)
#Function should return mean and median of randomly generated numbers as list
#Instructions
#Set seed. Use set.seed(1) to set seed
#Generate count number of random numbers between l_limit,u_limit. Hint Use - runif
#Round the numbers. Example : 1,2,3,..
#Find the mean and median
#Return as list. Title of elements should be mean,median
#Enter code below
random <- function(count,l_limit,u_limit){
set.seed(1)
b <- runif(count,l_limit,u_limit)
b <- round(b)
# print(b)
me <- mean(b)
md <- median(b)
p <- c(me,md)
# print(me)
# print(md)
lst <- list(me,md)
names(lst) <- c("mean","median")
# print(class(lst))
# return(p)
return(lst)
}
#End
random(100,0,50)
###############################
# Task 2: Write the function date which accepts three parameters (d,m,y) and return it in date format
# Instructions:
#Convert the values to type characters
#Concatenate the characters. Hint : Use paste function
#Return as date in format 'yyyy-mm-dd'
#Enter code below
date <- function(d,m,y){
dt <- paste(d,m,y)
dt <- as.Date(dt , '%d %m %Y')
return(dt)
}
#End
date(10,10,1999)
###############################
# Task 3: Write the function string_count which accepts a string as parameter and returns the count of words having more than 3 characters
#Instructions:
#Split the string. Use strsplit() to split the string
#Hint : Use length function to get the count of elements in list
#Hint : Use nchar function to find the number of characters in the string
#Return the count
#Enter code below
string_count <- function(s){
t <strsplit(s, split = " ")[[1]]
res <- c()
for(i in 1:length(t)){
# print(i)
# print(t[i])
len <- length(t[i])
# word_len <- length(strsplit(t[i], split = "")[[1]])
# print(word_len)
word_len <- nchar(t[i])
# print(word_len)
# print(len)
if(word_len>3){
res <- append(res, t[i])
}
}
return(length(res))
}
#End
string_count("It was well after noon when Sam came up the narrow lane behind the Parker place")